堆栈数据结构在 Javascript 中
在本文中,我们将讨论 Javascript 中的堆栈数据结构。
堆栈是一种抽象数据类型 (ADT),通常用在大多数编程语言中。称其为堆栈,因为它就像现实世界的堆栈,例如:一叠卡片或一堆盘子等。
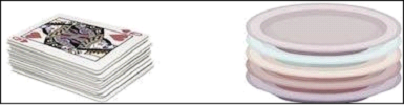
堆栈只允许一端进行操作。此特性使其成为后进先出数据结构。LIFO 代表后进先出。在此,最后放置(插入或添加)的元素,被首先访问。在堆栈术语中,插入操作称为 PUSH 操作,而删除操作称为 POP 操作。
以下图表显示了堆栈的操作:-
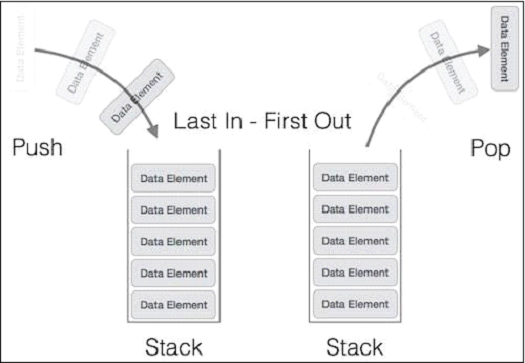
示例 1
以下示例演示了 Javascript 中的堆栈数据结构及其各种操作(push()、pop()、peek()、isEmpty()、isFull())。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Stack Data Structure</title> </head> <body> <script type="text/javascript"> class Stack { constructor() { this.stkArr = []; } // add element to the stack add(element) { return this.stkArr.push(element); } // remove element from the stack remove() { if (this.stkArr.length > 0) { document.write("<br>"); return "The Popped element is : " + this.stkArr.pop(); } } // view the last element peek() { document.write("<br>"); return ( "The Peek element of the stack is : " + this.stkArr[this.stkArr.length - 1] ); } // check if the stack is empty isEmpty() { document.write("<br>"); return this.stkArr.length == 0; } // the size of the stack size() { document.write("<br>"); return "The size of the stack is : " + this.stkArr.length; } display() { if (this.stkArr.length !== 0) { return "The stack elements are : " + this.stkArr + "<br>"; } else { document.write("The Stack is Empty..! <br>"); } } // empty the stack clear() { document.write("
The stack is cleared..!" + "<br>"); this.stkArr = []; } } let stack = new Stack(); stack.add(1); stack.add(2); stack.add(3); stack.add(4); document.write(stack.display()); stack.clear(); stack.display(); </script> </body> </html>
示例 2
在这个示例中,我们创建一个堆栈并使用 push() 操作向其中添加元素;并在清除堆栈前和清除堆栈后显示它。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Stack Data Structure</title> </head> <body> <script type="text/javascript"> class Stack { constructor() { this.stkArr = []; } // add element to the stack add(element) { return this.stkArr.push(element); } // remove element from the stack remove() { if (this.stkArr.length > 0) { document.write("<br>"); return "The Popped element is : " + this.stkArr.pop(); } } // view the last element peek() { document.write("<br>"); return ( "The Peek element of the stack is : " + this.stkArr[this.stkArr.length - 1] ); } // check if the stack is empty isEmpty() { document.write("<br>"); return this.stkArr.length == 0; } // the size of the stack size() { document.write("<br>"); return "The size of the stack is : " + this.stkArr.length; } display() { if (this.stkArr.length !== 0) { return "The stack elements are : " + this.stkArr + "<br>"; } else { document.write("The Stack is Empty..! <br>"); } } // empty the stack clear() { document.write("
The stack is cleared..!" + "<br>"); this.stkArr = []; } } let stack = new Stack(); stack.add(1); stack.add(2); stack.add(3); stack.add(4); document.write(stack.display()); document.write(stack.peek()); document.write(stack.size()); </script> </body> </html>
示例 3
以下是表示堆栈的完整 Javascript 类:-
class Stack { constructor(maxSize) { // Set default max size if not provided if (isNaN(maxSize)) { maxSize = 10; } this.maxSize = maxSize; // Init an array that'll contain the stack values. this.container = []; } display() { console.log(this.container); } isEmpty() { return this.container.length === 0; } isFull() { return this.container.length >= this.maxSize; } push(element) { // Check if stack is full if (this.isFull()) { console.log("Stack Overflow!") return; } this.container.push(element) } pop() { // Check if empty if (this.isEmpty()) { console.log("Stack Underflow!") return; } this.container.pop() } peek() { if (isEmpty()) { console.log("Stack Underflow!"); return; } return this.container[this.container.length - 1]; } clear() { this.container = []; } } const person = new Stack(10); person.push(10); person.push(44); person.push(55); person.display();
广告