使用MATLAB估计噪声图像中的高斯噪声
在数字图像中,高斯噪声是一种服从正态分布或高斯分布的噪声。在数字图像中,高斯噪声表现为像素强度值的随机变化。高斯噪声的估计是数字图像处理中一项重要的任务,用于执行去噪、图像恢复等各种操作。
数字图像中高斯噪声的估计方法
我们可以使用多种方法来估计数字图像中的高斯噪声。以下是几种常用的高斯噪声估计方法。
标准差法
直方图分析法
让我们通过示例详细讨论这两种方法。
使用标准差法估计高斯噪声
在数字图像处理中,标准差法是估计高斯噪声最简单的方法。它通过查找像素强度值的标准差来估计图像中的高斯噪声。
下面解释了使用标准差法估计数字图像中高斯噪声的分步过程。
步骤(1) - 读取包含高斯噪声的输入图像。
步骤(2) - 根据需要将图像转换为灰度图像。
步骤(3) - 将图像转换为双精度数据类型以进行计算。
步骤(4) - 通过计算图像像素强度的标准差来估计高斯噪声。
步骤(5) - 显示估计的高斯噪声。
步骤(6) - 显示噪声图像和去噪图像,以便进行视觉分析。
在MATLAB中,通过标准差法估计高斯噪声是一个六步的简单方法。
示例
让我们举个例子来了解使用MATLAB估计高斯噪声的代码实现。
% MATLAB code to estimate gaussian noise using standard deviation method % Read the noisy image noisy_img = imread('Noisy_Image.jpg'); %Convert the input noisy image to grayscale gray_img = rgb2gray(noisy_img); % Convert the gray image to double data type gray_img = im2double(gray_img); % Estimate the Gaussian noise using standard deviation estimated_noise = std2(gray_img); % Display the estimated gaussian noise in the image disp(['Estimated Gaussian Noise in the Image is: ' num2str(estimated_noise)]); % Remove the noise from the image denoised_img = imgaussfilt(gray_img, 2); % Display the noisy and denoised images figure; subplot(1, 2, 1); imshow(gray_img); title('Noisy Image'); subplot(1, 2, 2); imshow(denoised_img); title('Denoised Image');
输出
Estimated Gaussian Noise in the Image is: 0.25054
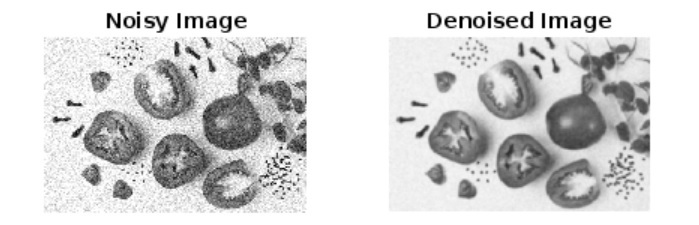
解释
在这个MATLAB示例中,我们使用标准差法估计了图像中的高斯噪声。
在代码中,我们首先使用“imread”函数读取噪声图像。然后,我们分别使用“rgb2gray”和“im2double”函数将输入图像转换为灰度图像和双精度数据类型以进行计算。
之后,我们使用“std2”函数估计图像中的高斯噪声。此函数计算噪声图像的像素强度值的标准差。
接下来,我们使用“disp”函数显示图像中估计的高斯噪声。
在下一步中,我们还使用高斯滤波器去除高斯噪声,并显示噪声图像和去噪图像以进行视觉比较。
这就是我们如何在MATLAB中使用标准差法估计高斯噪声的方法。
使用直方图分析法估计高斯噪声
在MATLAB中,我们可以通过分析图像的直方图来估计数字图像中的高斯噪声。在这种方法中,我们分析图像中像素强度值的分布。然后,我们可以使用这些估计值来对图像进行去噪。
下面解释了使用直方图分析法估计图像中高斯噪声的步骤。
步骤(1) - 使用“imread”函数读取噪声图像。
步骤(2) - 将输入图像转换为灰度图像以进行直方图分析。
步骤(3) - 计算并显示图像的直方图。
步骤(4) - 拟合噪声图像的高斯分布。
步骤(5) - 确定噪声图像拟合高斯分布的均值和标准差。
步骤(6) - 显示估计的高斯噪声,即噪声图像的均值和标准差。
步骤(7) - 对图像进行去噪。
步骤(8) - 显示噪声图像和去噪图像以进行视觉比较。
示例
让我们举个例子来了解这些步骤在估计图像中高斯噪声时的实现。
% MATLAB code to estimate gaussian noise using histogram analysis % Read the noisy image noisy_img = imread('noisy_image.jpg'); % Convert to the noisy image to grayscale gray_img = rgb2gray(noisy_img); % Compute the histogram of the noisy image [counts, binLocations] = imhist(gray_img); % Display the histogram of noisy image figure; bar(binLocations, counts); title('Histogram of Noisy Image'); xlabel('Pixel Intensity'); ylabel('Frequency'); % Fit gaussian distribution to pixel intensity values g = fitdist(gray_img(:), 'Normal'); % Estimate the mean value and standard deviation mean_value = g.mu; std_dev = g.sigma; % Display estimated gaussian noise parameters fprintf('Estimated Mean Value: %.2f
', mean_value); fprintf('Estimated Standard Deviation: %.2f
', std_dev); % Denoise the image using the gaussian filter denoised_img = imgaussfilt(gray_img, 1.5); % Display the noisy and denoised images figure; subplot(1, 2, 1); imshow(noisy_img); title('Noisy Image'); subplot(1, 2, 2); imshow(denoised_img, []); title('Denoised Image');
输出
运行此代码后,将产生以下输出-
直方图 -
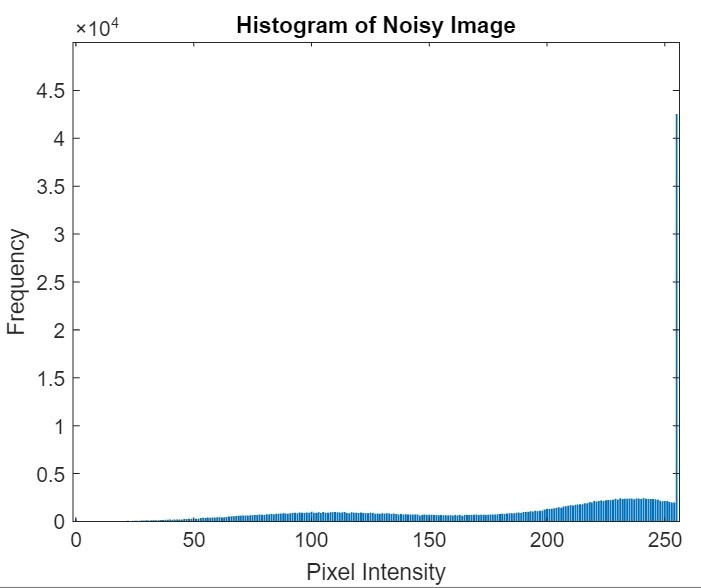
噪声参数 -
Estimated Mean Value: 187.94 Estimated Standard Deviation: 12.03
噪声图像和去噪图像 -
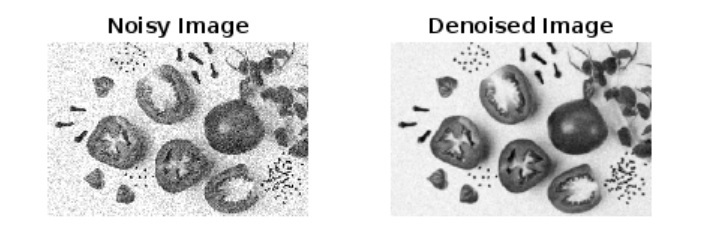
此示例演示了使用直方图分析法逐步骤估计图像中高斯噪声的过程。
结论
这就是关于使用MATLAB估计噪声图像中高斯噪声的所有内容。高斯噪声的估计是数字图像处理中的一项关键任务。它有助于对噪声图像进行去噪。MATLAB提供了多种方法来估计噪声图像中的高斯噪声。但最常用的方法是标准差法和直方图分析法。在本教程中,我通过MATLAB编程示例详细解释了这两种方法。