基于 Tkinter 在特定文本行中根据关键词突出显示颜色
创建有效的 GUI 通常涉及显示和操作文本。Tkinter 是流行的 Python GUI 工具包,它提供了一个通用的 Text 组件来处理基于文本的内容。在本教程中,我们将展示如何在 Tkinter 应用程序中根据特定条件突出显示特定文本行。
设置 Tkinter 环境
让我们从设置基本的 Tkinter 环境开始。确保已安装 Tkinter,方法是运行 -
pip install tk
创建简单的 Tkinter 窗口
现在,让我们创建一个带有 Text 组件的简单 Tkinter 窗口 -
import tkinter as tk # Create the Tkinter window root = tk.Tk() root.title("Highlighting colour for a specific line of text") root.geometry("720x250") # Create a Text widget text_widget = tk.Text(root, wrap=tk.WORD) text_widget.pack(expand=True, fill=tk.BOTH) # Start the Tkinter event loop root.mainloop()
这构成了我们文本突出显示演示的基础。
Tkinter 中的文本突出显示
要根据关键词突出显示特定行,我们将创建一个名为 highlight_keyword 的函数。此函数将与 Text 组件交互,将标签应用于文本的相关部分 -
def highlight_keyword(text_widget, keyword, line_number, fg_color='yellow', bg_color='red'): start_index = text_widget.index(f'{line_number}.0') end_index = text_widget.index(f'{line_number + 1}.0') line_text = text_widget.get(start_index, end_index) if keyword in line_text: start_index = start_index + '+%dc' % line_text.index(keyword) end_index = start_index + '+%dc' % len(keyword) text_widget.tag_add('highlight', start_index, end_index) text_widget.tag_config('highlight', foreground=fg_color, background=bg_color)
分解 highlight_keyword 函数
确定行索引 - 在 Text 组件中获取指定行的起始和结束索引。
检索行文本 - 提取指定行的文本内容。
检查关键词是否存在 - 验证该行中是否存在关键词。
应用标签 - 如果找到关键词,则计算关键词在该行中的索引,并将标签('highlight')应用于该范围。
配置标签样式 - 自定义标签的外观,指定前景色和背景色。
在 Tkinter 中结合文本插入和关键词突出显示
现在,让我们将文本插入和关键词突出显示结合到我们的 Tkinter 环境中 -
# Insert some text into the Text widget text_widget.insert(tk.END, "This is the first line.\n") text_widget.insert(tk.END, "This line contains the keyword.\n") text_widget.insert(tk.END, "This is the third line.\n") text_widget.insert(tk.END, "This is the fourth line.\n") text_widget.insert(tk.END, "This is the fifth line.\n") text_widget.insert(tk.END, "This is the sixth line.\n") # Highlight the line containing the keyword highlight_keyword(text_widget, 'keyword', 2)
将所有内容整合在一起
让我们将所有这些整合在一起并检查输出 -
示例
import tkinter as tk def highlight_keyword( text_widget, keyword, line_number, fg_color='yellow', bg_color='red'): start_index = text_widget.index(f'{line_number}.0') end_index = text_widget.index(f'{line_number + 1}.0') line_text = text_widget.get(start_index, end_index) if keyword in line_text: start_index = start_index + '+%dc' % line_text.index(keyword) end_index = start_index + '+%dc' % len(keyword) text_widget.tag_add('highlight', start_index, end_index) text_widget.tag_config( 'highlight', foreground=fg_color, background=bg_color ) # Create the Tkinter window root = tk.Tk() root.title("Highlighting colour for a specific line of text") root.geometry("720x250") # Create a Text widget text_widget = tk.Text(root, wrap=tk.WORD) text_widget.pack(expand=True, fill=tk.BOTH) # Insert some text into the Text widget text_widget.insert(tk.END, "This is the first line.\n") text_widget.insert(tk.END, "This line contains the keyword.\n") text_widget.insert(tk.END, "This is the third line.\n") text_widget.insert(tk.END, "This is the fourth line.\n") text_widget.insert(tk.END, "This is the fifth line.\n") text_widget.insert(tk.END, "This is the sixth line.\n") # Highlight the line containing the keyword highlight_keyword(text_widget, 'keyword', 2) # Start the Tkinter event loop root.mainloop()
这里,六行文本被插入到 Text 组件中。然后调用 highlight_keyword 函数来突出显示包含关键词“keyword”的行。
输出
运行此代码后,您将获得以下输出窗口 -
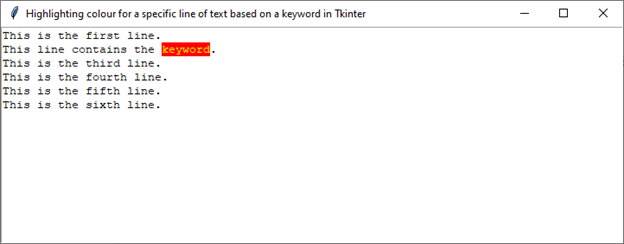
结论
Tkinter 中的文本突出显示增强了图形应用程序的可用性。使用 Text 组件和标记机制,开发人员可以根据各种条件实现动态且视觉上吸引人的文本突出显示。
广告