使用 Python 将文件移动到以创建和修改日期命名的目录
在本问题陈述中,我们必须借助 Python 将文件夹中的所有文件移动到以创建和修改日期命名的目录中。在此任务中,我们将使用 Python 的一些预定义库来平滑地处理文件重新定位。
理解问题
正如我们所见,根据日期或修改和创建时间来管理文件。因此,在本文中,我们将通过创建 Python 脚本简化此任务,该脚本将根据创建和修改日期移动文件,并将文件夹名称作为修改或创建日期。因此,基本上我们将查找该文件夹并移动文件,如果不存在根据日期命名的文件夹,我们将创建一个新文件夹,并根据文件的修改日期命名它,并将这些文件按创建或修改日期排序移动。
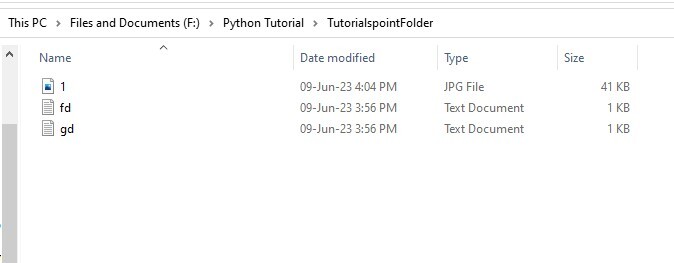
在上图中,我们可以看到这些文件及其修改日期。因此,我们将这些文件移动到修改日期文件夹中以对其进行整理。
算法
步骤 1 − 首先在 Python 脚本的开头导入必要的库。在我们的程序中,我们正在导入 OS、shutil 和 datetime 库。
步骤 2 − 定义存在文件的源文件路径以执行移动操作。
步骤 3 − 然后将当前工作目录更改为源目录。然后列出当前目录中的所有目录和文件。
步骤 4 − 因此,我们将需要一个工作文件夹,因此我们将使用 os 库的 getcwd 函数。
步骤 5 − 现在我们将使用 for 循环遍历当前工作目录中的所有文件。
步骤 6 − 在循环内部,我们将当前目录路径与文件或目录名称连接起来。
步骤 7 − 检查条件,如果我们处于正确的路径上,则将所有文件移动到当前目录。并在文件移动后删除目录。
步骤 8 − 如果未找到正确的路径,则获取文件的修改日期并创建目录,并将其命名为修改日期。并将所有文件移动到目标文件夹。
示例
import os as system import shutil import datetime source_directory = r"F:/Python Tutorial/Tutorialspoint folder/test" # Change the current working directory to the source directory system.chdir(source_directory) # List all directories and files in the current directory all_files = system.listdir() # need the current working folder output = system.getcwd() # Loop over each file in the current folder for dir_or_file in all_files: try: # Join the current directory path with the file/directory name path = system.path.join(output, dir_or_file) if system.path.isdir(path): # move directory's files to the current directory dir_files = system.listdir(path) for dir_file in dir_files: file_path = system.path.join(path, dir_file) shutil.move(file_path, output) # When the files are moved so remove the directory shutil.rmtree(path) else: # Get file's modification date modify_time = system.path.getmtime(path) modify_date = datetime.datetime.fromtimestamp(modify_time) # Create the directory as per the modification date dir_name = modify_date.strftime("%b-%d-%Y") dest = system.path.join(output, dir_name) # If directory is not present the Create it system.makedirs(dest, exist_ok=True) # Move the file to the destination folder shutil.move(path, dest) except Exception as e: print(f"Error occurred: {e}") print("Successfully moved the files.")
输出
$$\mathrm{移动前}$$
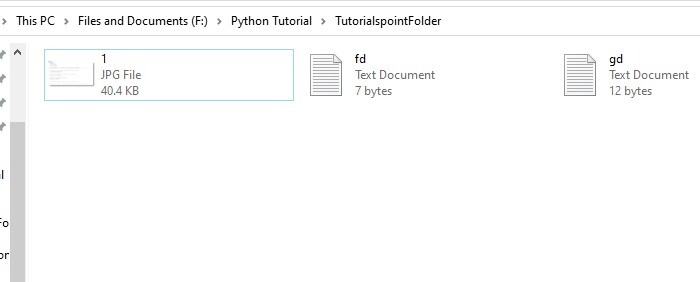
$$\mathrm{移动后}$$
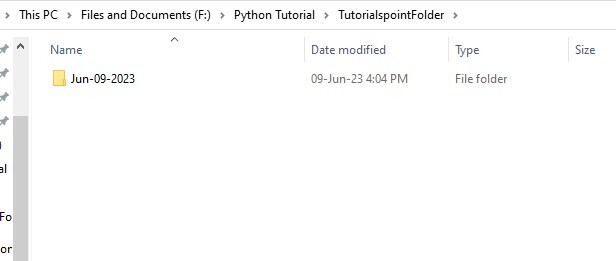
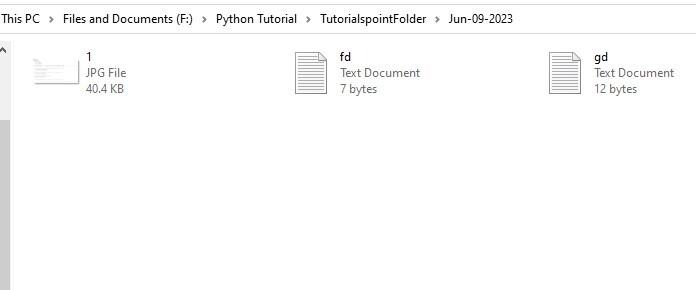
结论
因此,我们创建了一个代码,可以使用 Python 将所有文件移动到以创建和修改日期命名的目录中。因此,基本上我们使用了 Python 的 os、shutil 和 datetime 库。我们遍历了源路径中的所有文件和目录,并将字段移动到相应的目录。