Android 如何为特定文件扩展名设置 Intent 过滤器?
简介
Android Intent 过滤器是 Android 操作系统的重要组成部分。它们用于允许应用程序响应某些系统事件,例如启动 Activity 或接收广播。除了能够启动 Activity 外,Intent 过滤器还可以用于过滤特定文件类型。
特定文件类型的 Intent 过滤器是一种过滤器,它将导致应用程序响应用户打开具有特定扩展名的文件的请求。例如,当用户在文件管理器中点击 .txt 文件时,Android 操作系统将查询已安装应用程序列表,以查看是否有任何应用程序具有与请求的文件类型匹配的 Intent 过滤器。如果有,它将启动具有 Intent 过滤器的应用程序。在本文中,我们将了解如何为特定文件扩展名创建 Android Intent 过滤器。
实现
我们将创建一个简单的应用程序,在其中显示一个简单的 TextView,用于显示应用程序的标题。然后,我们将创建两个按钮。一个按钮用于查看,另一个按钮用于发送,点击此按钮时,我们将打开一个带有自定义参数的 Intent,这些参数将通过 Intent 过滤器传递。
步骤 1 - 在 Android Studio 中创建一个新项目。
导航到 Android Studio,如下面的屏幕所示。在下面的屏幕中,单击“新建项目”以创建一个新的 Android Studio 项目。
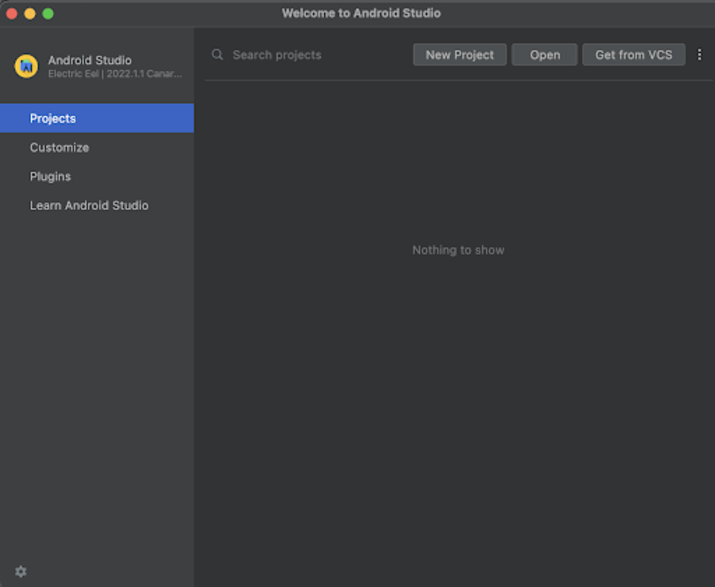
单击“新建项目”后,您将看到下面的屏幕。
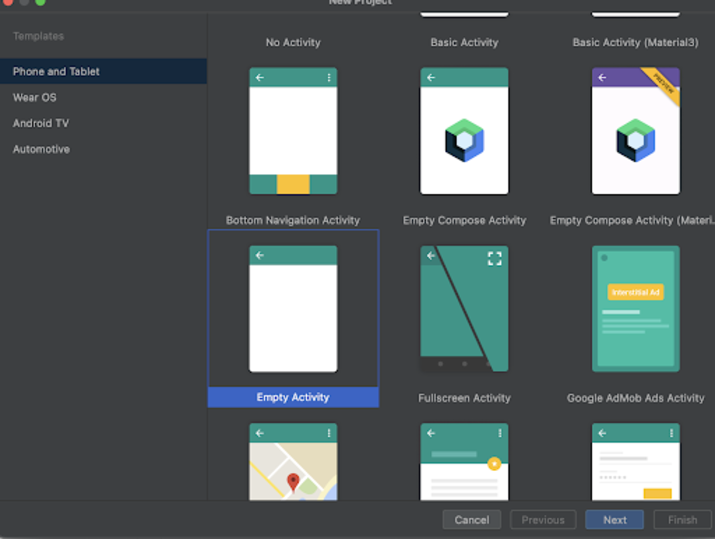
在此屏幕中,我们只需选择“空活动”并单击“下一步”。单击“下一步”后,您将看到下面的屏幕。
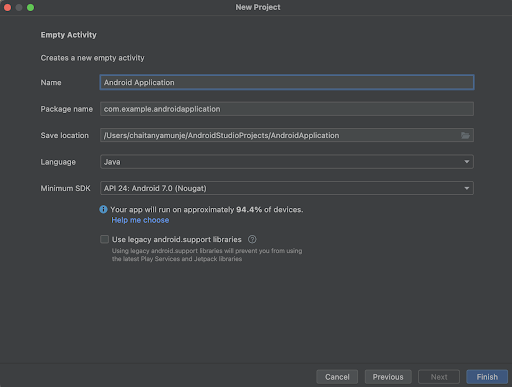
在此屏幕中,我们只需指定项目名称。然后包名称将自动生成。
注意 - 确保选择 Java 作为语言。
指定所有详细信息后,单击“完成”以创建一个新的 Android Studio 项目。
创建项目后,我们将看到两个打开的文件,即 activity_main.xml 和 MainActivity.java 文件。
步骤 3 - 使用 activity_main.xml。
导航到 activity_main.xml。如果此文件不可见。要打开此文件。在左侧窗格中导航到 app>res>layout>activity_main.xml 以打开此文件。打开此文件后。将以下代码添加到其中。代码中添加了注释以详细了解。
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/idRLLayout" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <!-- text view for displaying heading of the application --> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_above="@id/idBtnSendMsg" android:layout_centerInParent="true" android:padding="5dp" android:text="Intent Filter in Android" android:textAlignment="center" android:textAllCaps="false" android:textColor="@color/black" android:textSize="18sp" android:textStyle="bold" /> <!-- on below line we are creating a button to send message --> <Button android:id="@+id/idBtnSendMsg" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_centerInParent="true" android:layout_margin="20dp" android:text="Send Message" android:textAllCaps="false" /> <!-- on below line we are creating a button for view action --> <Button android:id="@+id/idBtnView" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_below="@id/idBtnSendMsg" android:layout_centerInParent="true" android:layout_margin="20dp" android:text="View Action" android:textAllCaps="false" /> </RelativeLayout>
说明 - 在上面的代码中,我们创建了一个 Relative Layout 作为根布局,并在其中创建了一个简单的 TextView,用于显示应用程序的标题。之后,我们创建了两个按钮,一个按钮用于发送操作,另一个按钮用于查看操作。
步骤 4 - 使用 AndroidManifest.xml 文件。
导航到 AndroidManifest.xml 文件并将以下代码添加到其中。代码中添加了注释以详细了解。
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools"> <application android:allowBackup="true" android:dataExtractionRules="@xml/data_extraction_rules" android:fullBackupContent="@xml/backup_rules" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:preserveLegacyExternalStorage="true" android:requestLegacyExternalStorage="true" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/Theme.AndroidJAVAApp" android:usesCleartextTraffic="true" tools:targetApi="31"> <activity android:name=".MainActivity" android:exported="true"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> <!-- on below line creating an intent filter for send action --> <intent-filter> <action android:name="android.intent.action.SEND" /> <category android:name="android.intent.category.DEFAULT" /> <data android:mimeType="text/plain" /> </intent-filter> <!-- on below line creating an intent filter for view action --> <intent-filter> <action android:name="android.intent.action.VIEW" /> <category android:name="android.intent.category.DEFAULT" /> <category android:name="android.intent.category.BROWSABLE" /> <data android:scheme="http" /> </intent-filter> </activity> </application> </manifest>
步骤 5 - 使用 MainActivity.java 文件。
导航到 MainActivity.java。如果此文件不可见。要打开此文件。在左侧窗格中导航到 app>res>layout>MainActivity.java 以打开此文件。打开此文件后。将以下代码添加到其中。代码中添加了注释以详细了解。
package com.example.androidjavaapp; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.Button; import androidx.appcompat.app.AppCompatActivity; public class MainActivity extends AppCompatActivity { // on below line creating a variable for button. private Button sendBtn, viewBtn; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // on below line we are initializing variables. sendBtn = findViewById(R.id.idBtnSendMsg); viewBtn = findViewById(R.id.idBtnView); // on below line we are adding click listener for send button. sendBtn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // on below line we are creating an intent for send action. Intent i = new Intent(Intent.ACTION_SEND); // on below line we are setting intent type and starting our activity. i.setType("text/plain"); i.putExtra(Intent.EXTRA_EMAIL, "niranjantest@gmail.com"); i.putExtra(Intent.EXTRA_SUBJECT, "This is a dummy message"); i.putExtra(Intent.EXTRA_TEXT, "Dummy test message"); startActivity(i); } }); viewBtn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // on below line we are creating an intent for view action. Intent i = new Intent(Intent.ACTION_VIEW); startActivity(i); } }); } }
说明 - 在上面的代码中,我们首先为按钮创建一个变量。之后,我们将看到一个 onCreate 方法。在此方法内部,我们将看到一个 setContentView 方法。此方法将从 activity_main.xml 文件加载 UI。之后,我们从我们在 activity_main.xml 文件中指定的 id 初始化按钮的变量。之后,我们为按钮添加了一个点击监听器。在按钮的点击监听器内部,我们通过为每个按钮指定两个操作来创建一个 Intent,然后通过调用 startActivity 方法打开此 Intent。
添加上述代码后,我们只需单击顶部栏中的绿色图标即可在移动设备上运行我们的应用程序。
注意 - 确保您已连接到您的真实设备或模拟器。
输出
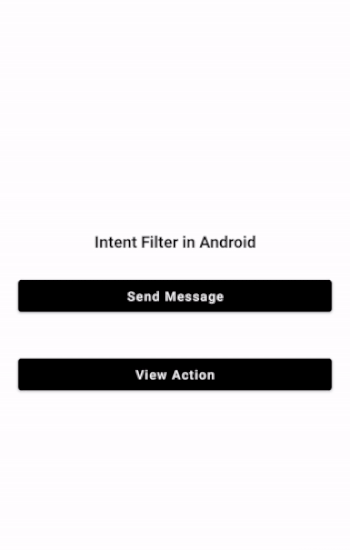
结论
在本文中,我们了解了 Android 中的 Intent 过滤器是什么,以及如何在 Android 应用程序中使用这些 Intent 过滤器来打开特定 Intent。