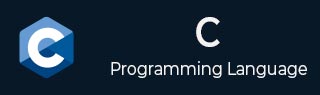
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - CMPLX() 函数
C 的complex 库CMPLX() 函数通常用于生成由其实部和虚部 (imag) 组成的复数。
如果实部和虚部为“float”类型,我们可以使用cmplxf()生成复数,并使用crealf()和cimagf()获取实部和虚部,对于长双精度型,使用cmplxl()、creall()和cimagl()。
此函数可用于科学和工程应用,包括信号处理、控制系统和量子力学。
语法
以下是CMPLX() 函数的 C 库语法:
double complex CMPLX( double real, double imag );
参数
此函数接受以下参数:
-
real - 表示复数的实部。它是双精度型。
-
imag - 表示复数的虚部 (imag)。它是双精度型。
返回值
此函数返回一个双精度型的复数。
示例 1
以下是一个基本的 C 程序,演示了如何使用CMPLX() 生成复数。
#include <stdio.h> #include <complex.h> int main() { double real = 3.0; double imag = 4.0; // Use the CMPLX function to create complex number double complex z = CMPLX(real, imag); printf("The complex number is: %.2f + %.2fi\n", creal(z), cimag(z)); return 0; }
输出
以下是输出:
The complex number is: 3.00 + 4.00i
示例 2
计算共轭复数
让我们看另一个例子,我们使用CMPLX() 函数创建复数,然后我们使用“conj()”计算复数的共轭复数。
#include <stdio.h> #include <complex.h> int main() { double real = 3.0; double imag = 4.0; // Use the CMPLX function to create complex number double complex z = CMPLX(real, imag); printf("The complex number is: %.2f + %.2fi\n", creal(z), cimag(z)); // Calculate conjugate double complex z_conjugate = conj(z); printf("The conjugate of the complex number is: %.2f + %.2fi\n", creal(z_conjugate), cimag(z_conjugate)); return 0; }
输出
以下是输出:
The complex number is: 3.00 + 4.00i The conjugate of the complex number is: 3.00 + -4.00i
示例 3
对复数执行算术运算
以下示例生成两个复数,并对这两个复数执行算术运算。
#include <stdio.h> #include <complex.h> int main() { double real1 = 2.0, imag1 = 3.0; double real2 = 4.0, imag2 = -5.0; // Use the CMPLX function double complex z1 = CMPLX(real1, imag1); double complex z2 = CMPLX(real2, imag2); // Arithmetic operations double complex sum = z1 + z2; double complex difference = z1 - z2; // Display the results of the operations printf("Complex number z1: %.2f + %.2fi\n", creal(z1), cimag(z1)); printf("Complex number z2: %.2f + %.2fi\n", creal(z2), cimag(z2)); printf("Sum: %.2f + %.2fi\n", creal(sum), cimag(sum)); printf("Difference: %.2f + %.2fi\n", creal(difference), cimag(difference)); return 0; }
输出
以下是输出:
Complex number z1: 2.00 + 3.00i Complex number z2: 4.00 + -5.00i Sum: 6.00 + -2.00i Difference: -2.00 + 8.00i
c_library_complex_h.htm
广告