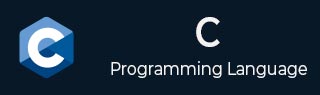
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - abs() 函数
C 的stdlib 库 abs() 函数用于返回指定数字的绝对值,其中绝对值表示正数。
此函数仅返回正整数。例如,如果我们有一个整数 -2,我们想要获取 -2 的绝对值。然后我们使用 abs() 函数返回正数 2。
语法
以下是 abs() 函数的 C 库语法 -
int abs(int x)
参数
此函数接受一个参数 -
x - 它表示一个整数值。
返回值
此函数返回整数的绝对值。
示例 1
在此示例中,我们创建一个基本的 C 程序来演示 abs() 函数的使用。
#include<stdio.h> #include<stdlib.h> int main(){ int x = -2, res; printf("Original value of X is %d\n", x); // use the abs() function to get the absolute value res = abs(x); printf("Absolute value of X is %d", res); }
输出
以下是输出 -
Original value of X is -2 Absolute value of X is 2
示例 2
让我们再创建一个示例来获取正整数和负整数的绝对值。使用 abs() 函数。
#include<stdio.h> #include<stdlib.h> int main(){ int x, y; //absolute of negative number x = abs(-10); printf("Absolute of -10: %d\n", x); // absolute of positive number y = abs(12); printf("Absolute of 12: %d", y); }
输出
以下是输出 -
Absolute of -10: 10 Absolute of 12: 12
示例 3
创建另一个 C 程序来获取指定整数的绝对值。使用 abs() 函数。
#include<stdio.h> #include<stdlib.h> int main(){ int x; //absolute of negative number x = abs(-10*100); printf("Absolute of -10*100: %d\n", x); }
输出
以下是输出 -
Absolute of -10*100: 1000
广告