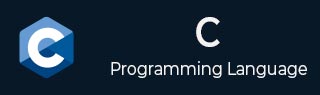
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - acos() 函数
C 库的 acos() 函数返回x的反余弦值(以弧度为单位)。
此参数必须在 -1 到 1 的范围内,如果给定的输入超出范围,它将返回 nan 并可能将 errno 设置为 EDOM。
语法
以下是 C 库 acos() 函数的语法:
double acos(double x)
参数
此函数只接受一个参数:
x - 这是区间 [-1,+1] 内的浮点值。
返回值
此函数返回 x 的主值反余弦,在区间 [0, pi] 弧度内,否则返回 nan(非数值)的值。
示例 1
以下是一个基本的 C 库程序,用于演示 acos() 函数。
#include <stdio.h> #include <math.h> #define PI 3.14159265 int main () { double x, ret, val; x = 0.9; val = 180.0 / PI; ret = acos(x) * val; printf("The arc cosine of %lf is %lf degrees", x, ret); return(0); }
输出
以上代码产生以下结果:
The arc cosine of 0.900000 is 25.855040 degrees
示例 2
以下程序说明了 acos() 的用法,其中参数 x > 1 或 x < -1,它将返回 nan 作为结果。
#include <stdio.h> #include <math.h> int main() { double x = 4.4, res; // Function call to calculate acos(x) value res = acos(x); printf("acos(4.4) = %f radians\n", res); printf("acos(4.4) = %f degrees\n", res * 180 / 3.141592); return 0; }
输出
执行以上代码后,我们将得到以下结果:
acos(4.4) = nan radians acos(4.4) = nan degrees
示例 3
在这里,我们设置宏在 1 到 -1 之间的数值范围内,以检查给定的数字是否存在于范围内。
#include <stdio.h> #include <stdlib.h> #include <math.h> #define MAX 1.0 #define MIN -1.0 int main(void) { double x = 10, y = -1; y = acos(x); if (x > MAX) printf( "Error: %lf not in the range!\n", x ); else if (x < MIN) printf( "Error: %lf not in the range!\n", x ); else printf("acos(%lf) = %lf\n", x, y); }
输出
执行以上代码后,我们将得到以下结果:
Error: 10.000000 not in the range!
广告