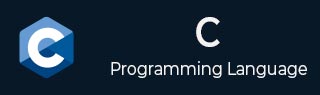
- C标准库
- C库 - 首页
- C库 - <assert.h>
- C库 - <complex.h>
- C库 - <ctype.h>
- C库 - <errno.h>
- C库 - <fenv.h>
- C库 - <float.h>
- C库 - <inttypes.h>
- C库 - <iso646.h>
- C库 - <limits.h>
- C库 - <locale.h>
- C库 - <math.h>
- C库 - <setjmp.h>
- C库 - <signal.h>
- C库 - <stdalign.h>
- C库 - <stdarg.h>
- C库 - <stdbool.h>
- C库 - <stddef.h>
- C库 - <stdio.h>
- C库 - <stdlib.h>
- C库 - <string.h>
- C库 - <tgmath.h>
- C库 - <time.h>
- C库 - <wctype.h>
C库 - atan() 函数
C语言math库的atan()函数用于返回传入参数'arg'的反正切值(反三角函数),结果以弧度表示,范围在[-π/2, +π/2]之间。
反正切,也称为反正切函数。它是正切函数的反函数,反转正切函数的作用。
语法
以下是C语言atan()函数的语法:
double atan( double arg );
参数
此函数接受单个参数:
-
arg − 表示一个浮点数。
返回值
如果没有错误发生,则返回参数 (arg) 的反正切值,结果以弧度表示,范围在 [-π/2, +π/2] 之间。如果由于下溢而发生范围错误,则返回正确的结果(舍入后)。
示例 1
以下是一个基本的C程序,演示了如何使用atan()获取以弧度表示的角度。
#include <stdio.h> #include <math.h> int main() { double arg = 1.0; double res = atan(arg); printf("The arc tangent of %f is %f radians.\n", arg, res); return 0; }
输出
以下是输出:
The arc tangent of 1.000000 is 0.785398 radians.
示例 2
让我们创建另一个示例,我们使用atan()函数来获取数组中每个元素的反正切值。
#include <stdio.h> #include <math.h> int main() { double arg[] = {10, 20, 25, 30}; int length = sizeof(arg) / sizeof(arg[0]); for(int i=0; i<length; i++){ double res = atan(arg[i]); printf("The arc tangent of %f is %f radians.\n", arg[i], res); } return 0; }
输出
以下是输出:
The arc tangent of 10.000000 is 1.471128 radians. The arc tangent of 20.000000 is 1.520838 radians. The arc tangent of 25.000000 is 1.530818 radians. The arc tangent of 30.000000 is 1.537475 radians.
示例 3
现在,创建一个另一个C程序来显示反正切值以度表示。
#include <stdio.h> #include <math.h> #define PI 3.14159265 int main () { double arg, res_in_degree, val; arg = 1.0; val = 180.0 / PI; res_in_degree = atan (arg) * val; printf("The arc tangent of %lf is %lf degrees", arg, res_in_degree); return(0); }
输出
以下是输出:
The arc tangent of 1.000000 is 45.000000 degrees
广告