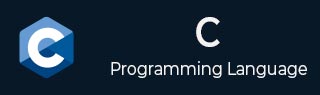
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - atexit() 函数
C 的stdlib 库 atexit() 函数会在程序正常终止时调用指定的函数“func”。我们可以在任何地方注册终止函数,但它将在程序终止时被调用。
当通过对 atexit() 函数的不同调用指定多个函数时,它们将按照栈的顺序执行。
此函数通常用于执行清理任务,例如在程序退出之前保存状态、释放资源或显示消息。
语法
以下是 atexit() 函数的 C 库语法:
int atatexit(void (*func)(void))
参数
此函数接受一个参数:
func - 它表示指向在程序正常终止时要调用的函数的指针。
返回值
此函数返回以下值:
- 零 - 如果函数注册成功。
- 非零 - 如果函数注册失败。
示例 1
在此示例中,我们创建一个基本的 C 程序来演示 atexit() 函数的使用。
#include <stdio.h> #include <stdlib.h> void tutorialspoint(void) { printf("tutorialspoint.com India\n"); } int main() { if (atexit(tutorialspoint) != 0) { fprintf(stderr, "Failed to register the tutorialspoint function with atexit\n"); return EXIT_FAILURE; } printf("This will be printed before the program exits.\n"); return EXIT_SUCCESS; }
输出
以下是输出:
This will be printed before the program exits. tutorialspoint.com India
示例 2
让我们创建一个另一个 C 程序,其中 atexit() 函数被多次调用。因此,所有指定的函数都按栈的顺序执行。
#include <stdio.h> #include <stdlib.h> // first function void first() { printf("Exit 1st \n"); } // second function void second() { printf("Exit 2nd \n"); } // third function void third() { printf("Exit 3rd \n"); } // main function int main() { int value1, value2, value3; value1 = atexit(first); value2 = atexit(second); value3 = atexit(third); if ((value1 != 0) || (value2 != 0) || (value3 != 0)) { printf("registration Failed for atexit() function. \n"); exit(1); } // it will execute at the first printf("Registration successful \n"); return 0; }
输出
以下是输出:
Registration successful Exit 3rd Exit 2nd Exit 1st
示例 3
在这里,我们创建了一个 C 程序,它执行两个数字的加法和减法,并使用 atexit() 以栈的方式显示这些函数的结果。
#include <stdio.h> #include <stdlib.h> // first function void add() { int a=10; int b=5; int res = a+b; printf("addition of two number is: %d\n", res); } // second function void sub() { int a=10; int b=5; int res = a-b; printf("subtraction of two number is: %d\n", res); } // main function int main() { int value1, value2; value1 = atexit(add); value2 = atexit(sub); if ((value1 != 0) || (value2 != 0)) { printf("registration Failed for atexit() function. \n"); exit(1); } // it will execute at the first printf("calculation successful \n"); return 0; }
输出
以下是输出:
calculation successful subtraction of two number is: 5 addition of two number is: 15
广告