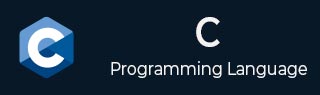
- C标准库
- C库 - 首页
- C库 - <assert.h>
- C库 - <complex.h>
- C库 - <ctype.h>
- C库 - <errno.h>
- C库 - <fenv.h>
- C库 - <float.h>
- C库 - <inttypes.h>
- C库 - <iso646.h>
- C库 - <limits.h>
- C库 - <locale.h>
- C库 - <math.h>
- C库 - <setjmp.h>
- C库 - <signal.h>
- C库 - <stdalign.h>
- C库 - <stdarg.h>
- C库 - <stdbool.h>
- C库 - <stddef.h>
- C库 - <stdio.h>
- C库 - <stdlib.h>
- C库 - <string.h>
- C库 - <tgmath.h>
- C库 - <time.h>
- C库 - <wctype.h>
C库 - atof() 函数
C 的stdlib 库 atof() 函数用于将字符串转换为浮点数,并将转换后的浮点数表示为其对应的双精度值。
浮点数是一种包含小数部分的整数类型,使用小数点表示。例如,像 10.05 和 5.5005 这样的数字是浮点数。
语法
以下是 atof() 函数的 C 库语法:
double atof(const char *str)
参数
此函数接受单个参数:
-
str − 它是一个指向以 null 结尾的字符串的指针,表示一个浮点数。
返回值
此函数返回一个与双精度表示相对应的浮点数。如果输入字符串不是有效的浮点数,则返回 0.0。
示例 1
以下是演示 atof() 函数用法的基本 C 示例。
#include <stdlib.h> #include <stdio.h> int main(void) { double res; char *str; str = "-1509.10E-10"; res = atof(str); printf("res = %.4e\n", res); }
输出
以下是输出:
res = -1.5091e-007
示例 2
在这个例子中,我们连接了两个字符串,然后使用 atof() 函数将结果字符串转换为浮点数。
#include <stdio.h> #include <stdlib.h> #include <string.h> int main() { // Define two strings to concatenate char str1[] = "123.456"; char str2[] = "789"; //calculate the length of string first + second int length = strlen(str1) + strlen(str2) + 1; // Allocate memory for the concatenated string char *concatenated = malloc(length); // check memory allocation if null return 1. if(concatenated == NULL) { printf("Memory allocation failed\n"); return 1; } // Concatenate str1 and str2 strcpy(concatenated, str1); strcat(concatenated, str2); // Convert concatenated string into a floating point number. // use the atof() function double number = atof(concatenated); printf("The concatenated string is: %s\n", concatenated); printf("The floating point number is: %f\n", number); // at the last free the alocated memory free(concatenated); return 0; }
输出
以下是输出:
The concatenated string is: 123.456789 The floating point number is: 123.456789
示例 3
以下是另一个示例,我们在这里将数字字符串和字符字符串都转换为浮点数。
#include <stdio.h> #include <stdlib.h> #include <string.h> int main () { float res; char str[20]; //define a string strcpy(str, "151413.10e"); //convert into float //use atof() function res = atof(str); printf("String value = %s\n" , str); printf("Float value = %f\n", res); strcpy(str, "tutorialspoint.com"); //use atof() function res = atof(str); printf("String value = %s\n", str); printf("Float value = %f\n", res); return(0); }
输出
以下是输出:
String value = 151413.10e Float value = 151413.093750 String value = tutorialspoint.com Float value = 0.000000
广告