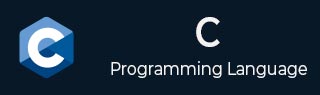
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - atol() 函数
C 的stdlib 库 atol() 函数用于将作为参数传递的字符串转换为长整型数字。例如,如果我们有字符串“123”,并且我们想将其转换为长整型 123,则可以使用 atol("123")。
此函数首先跳过任何前导空白字符,然后将后续字符作为数字的一部分进行处理。如果字符串以“+”或“-”号开头,则将其视为数字。当找到无效字符串时,转换停止。
语法
以下是 atol() 函数的 C 库语法:
long int atol(const char *str)
参数
此函数接受单个参数:
-
str − 它是一个指向空终止字符串的指针,表示一个长整型。
返回值
此函数返回一个长整型数字,表示长整型值。如果输入字符串不是有效的数字字符串,则返回 0。
示例 1
以下是一个基本的 C 程序,它使用 atol() 函数将字符串值转换为长整型值。
#include <stdlib.h> #include <stdio.h> int main(void) { long int val; char *str; str = "20152030"; //convert string into long int val = atol(str); printf("Long int value = %ld", val); }
输出
以下是输出:
Long int value = 20152030
示例 2
让我们来看另一个 C 程序,我们将两个字符串连接起来,然后使用 atol() 函数将结果字符串转换为长整型数字。
#include <stdio.h> #include <stdlib.h> #include <string.h> int main() { // Define two strings to concatenate char str1[] = "24"; char str2[] = "2024"; //calculate the length of string first + second int length = strlen(str1) + strlen(str2) + 1; // Allocate memory for the concatenated string char *concatenated = malloc(length); // check memory allocation if null return 1. if(concatenated == NULL) { printf("Memory allocation failed\n"); return 1; } // Concatenate str1 and str2 strcpy(concatenated, str1); strcat(concatenated, str2); // Convert concatenated string into a long int. // use the atol() function long int number = atol(concatenated); printf("The concatenated string is: %s\n", concatenated); printf("The long int value is: %ld\n", number); // at the last free the alocated memory free(concatenated); return 0; }
输出
以下是输出:
The concatenated string is: 242024 The long int value is: 242024
示例 3
下面的 C 程序将字符字符串转换为长整型数字。
#include <stdio.h> #include <stdlib.h> #include <string.h> int main () { long int res; char str[20]; //define a string strcpy(str, "tutorialspointIndia"); //use atoi() function res = atol(str); printf("String Value = %s\n", str); printf("Integral Number = %ld\n", res); return(0); }
输出
以下是输出:
String Value = tutorialspointIndia Integral Number = 0
广告