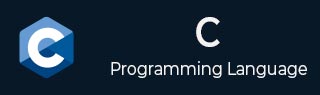
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - bsearch() 函数
C 的stdlib 库 bsearch() 函数对已排序的数组执行二分查找。它用于在数组中查找特定元素。此函数搜索由 base 指向的 n 个对象的数组。它查找与 key 指向的对象匹配的元素。
二分查找是一种搜索算法,用于在已排序的数组中查找目标值的位置。
语法
以下是 bsearch() 函数的 C 库语法:
void *bsearch(const void *key, const void *base, size_t nitems, size_t size, int (*compar)(const void *, const void *))
参数
此函数接受以下参数:
key - 它表示需要搜索的元素的指针。
base - 它表示数组第一个元素的指针。
nitems - 它表示数组中的元素数量。
size - 它表示数组中每个元素的大小。
compare - 它表示一个比较两个元素的函数。
返回值
此函数返回指向数组中匹配元素的指针。否则,它返回 NULL 指针。
示例 1
在此示例中,我们创建一个基本的 C 程序来演示 bsearch() 函数的使用。
#include <stdio.h> #include <stdlib.h> int compare(const void * a, const void * b) { return ( *(int*)a - *(int*)b ); } // main function int main () { int values[] = { 5, 20, 10, 30, 40 }; int *item; int key = 30; /* using bsearch() to find value 30 in the array */ item = (int*) bsearch (&key, values, 5, sizeof (int), compare); if( item != NULL ) { printf("Found item = %d\n", *item); } else { printf("Item = %d could not be found\n", *item); } return(0); }
输出
以下是输出:
Found item = 30
示例 2
下面的 C 程序使用 bsearch() 函数在已排序的数组中搜索字符。
#include <stdio.h> #include <stdlib.h> // Comparison function int comfunc(const void* a, const void* b) { return (*(char*)a - *(char*)b); } // main function int main() { // sorted array char arr[] = {'a', 'b', 'c', 'd', 'e'}; int n = sizeof(arr) / sizeof(arr[0]); // searching key char key = 'c'; // using bsearch() char* item = (char*)bsearch(&key, arr, n, sizeof(char), comfunc); // If the key is found, print its value and index if (item != NULL) { printf("'%c' Found at index %ld\n", *item, item - arr); } else { printf("Character '%c' is not found\n", key); } return 0; }
输出
以下是输出:
'c' Found at index 2
示例 3
以下是一个示例,我们创建了一个 C 程序来使用 bsearch() 函数在已排序的数组中搜索字符串。
#include <stdio.h> #include <stdlib.h> #include <string.h> // Comparison function int comfunc(const void* a, const void* b) { return strcmp(*(const char**)a, *(const char**)b); } // main function int main() { // Sorted array of strings const char* arr[] = {"Aman", "Shriansh", "Tapas", "Vivek"}; int n = sizeof(arr) / sizeof(arr[0]); // Searching key const char* key = "Aman"; // Using bsearch() const char** item; item = (const char**)bsearch(&key, arr, n, sizeof(const char*), comfunc); // If the key is found, print its value and index if (item != NULL) { printf("'%s' Found at index %ld\n", *item, item - arr); } else { printf("Name '%s' is not found\n", key); } return 0; }
输出
以下是输出:
'Aman' Found at index 0
广告