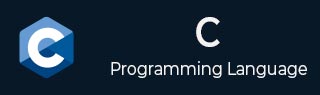
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - calloc() 函数
C 的stdlib 库calloc() 函数用于分配请求的内存并返回指向它的指针。它为对象数组预留一块内存,并将分配的存储区中的所有字节初始化为零。
如果我们尝试在没有初始化的情况下读取已分配内存的值,我们将得到“零”,因为 calloc() 已经将其初始化为 0。
语法
以下是 calloc() 函数的 C 库语法 -
void *calloc(size_t nitems, size_t size)
参数
此函数接受以下参数 -
-
nitems - 它表示要分配的元素数量。
-
size - 它表示每个元素的大小。
返回值
以下是返回值 -
-
返回指向已分配内存的指针,所有字节都初始化为零。
-
如果分配失败,则返回空指针。
示例 1
在此示例中,我们创建了一个基本的 C 程序来演示如何使用 calloc() 函数。
#include <stdio.h> #include <stdlib.h> int main() { int n = 5; int *array; // use calloc function to allocate the memory array = (int*)calloc(n, sizeof(int)); if (array == NULL) { fprintf(stderr, "Memory allocation failed!\n"); return 1; } //Display the array value printf("Array elements after calloc: "); for (int i = 0; i < n; i++) { printf("%d ", array[i]); } printf("\n"); //free the allocated memory free(array); return 0; }
输出
以下是输出 -
Array elements after calloc: 0 0 0 0 0
示例 2
让我们再创建一个 C 示例,我们使用 calloc() 函数为数字分配内存。
#include <stdio.h> #include <stdlib.h> int main() { long *number; //initialize number with null number = NULL; if( number != NULL ) { printf( "Allocated 10 long integers.\n" ); } else { printf( "Can't allocate memory.\n" ); } //allocating memory of a number number = (long *)calloc( 10, sizeof( long ) ); if( number != NULL ) { printf( "Allocated 10 long integers.\n" ); } else { printf( "\nCan't allocate memory.\n" ); } //free the allocated memory free( number ); }
输出
以下是输出 -
Can't allocate memory. Allocated 10 long integers.
示例 3
以下是使用 calloc() 函数为指针分配内存的 C 程序,大小为零。
#include <stdio.h> #include <stdlib.h> int main() { int *pointer = (int *)calloc(0, 0); if (pointer == NULL) { printf("Null pointer \n"); } else { printf("Address = %p", (void*)pointer); } free(pointer); return 0; }
输出
以下是输出 -
Address = 0x55c5f4ae02a0
广告