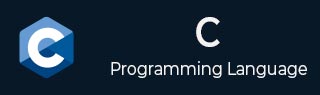
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - clock() 函数
C 库的 clock() 函数返回程序启动以来经过的时钟滴答数。要获取 CPU 使用的秒数,我们需要除以 CLOCKS_PER_SEC。在 CLOCKS_PER_SEC 等于 1000000 的 32 位系统上,此函数大约每 72 分钟返回相同的值。
CLOCKS_PER_SEC 是一种用于构建时间程序的宏。
语法
以下是 C 库 clock() 函数的语法:
clock_t clock(void)
参数
- 此函数不接受任何参数。
返回值
此函数返回程序启动以来经过的时钟滴答数。如果失败,则返回 -1。
示例 1
以下是一个基本的 C 库程序,用于演示 clock() 函数。
#include <time.h> #include <stdio.h> int main () { clock_t start_t, end_t; double total_t; int i; start_t = clock(); printf("Starting of the program, start_t = %ld\n", start_t); printf("Going to scan a big loop, start_t = %ld\n", start_t); for(i=0; i< 10000000; i++) { } end_t = clock(); printf("End of the big loop, end_t = %ld\n", end_t); total_t = (double)(end_t - start_t) / CLOCKS_PER_SEC; printf("Total time taken by CPU: %f\n", total_t ); printf("Exiting of the program...\n"); return(0); }
以上代码产生以下结果:
Starting of the program, start_t = 1194 Going to scan a big loop, start_t = 1194 End of the big loop, end_t = 26796 Total time taken by CPU: 0.025602 Exiting of the program...
示例 2
在本例中,我们使用 clock() 函数演示了各种操作的处理器时间(以秒为单位)的测量。
#include <stdio.h> #include <time.h> #include <math.h> int main() { float a; clock_t time_req; // Measure time for multiplication time_req = clock(); for (int i = 0; i < 200000; i++) { a = log(i * i * i * i); } time_req = clock() - time_req; printf("Processor time taken for multiplication: %f seconds\n", (float)time_req / CLOCKS_PER_SEC); // Measure time using pow function time_req = clock(); for (int i = 0; i < 200000; i++) { a = log(pow(i, 4)); } time_req = clock() - time_req; printf("Processor time taken in pow function: %f seconds\n", (float)time_req / CLOCKS_PER_SEC); return 0; }
输出
执行以上代码后,我们得到以下结果:
Processor time taken for multiplication: 0.005235 seconds Processor time taken in pow function: 0.010727 seconds
广告