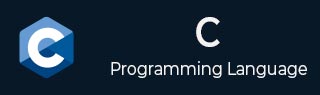
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - exit() 函数
C 的stdlib 库exit() 函数允许我们立即终止或退出调用进程。exit() 函数关闭属于进程的所有打开的文件描述符,并且进程的任何子进程都将由进程 1(init)继承,并且进程父进程将收到 SIGCHLD 信号。
“SIGCHLD”信号用于管理子进程,允许父进程处理其终止并收集退出状态。
语法
以下是exit() 函数的 C 库语法 -
void exit(int status)
参数
此函数接受一个参数 -
status - 它表示返回给父进程的状态值或退出代码。
- 0 或 EXIT_SUCCESS:0 或 EXIT_SUCCESS 表示程序已成功执行,未遇到任何错误。
- 1 或 EXIT_FAILURE:1 或 EXIT_FAILURE 表示程序遇到错误,无法成功执行。
返回值
此函数不返回值。
示例 1:带有状态“EXIT_FAILURE”的 exit()
让我们创建一个基本的 c 程序来演示 exit() 函数的使用。
#include <stdio.h> #include <stdlib.h> int main() { FILE *fp; printf("Opening the tutorialspoint.txt\n"); // open the file in read mode fp = fopen("tutorialspoint.txt", "r"); if (fp == NULL) { printf("Error opening file\n"); // Terminate the program with an error code exit(EXIT_FAILURE); } fclose(fp); // Terminate the program successfully exit(EXIT_SUCCESS); }
输出
以下是输出 -
Open the tutorialspoint.txt Error opening file
示例 2
在此示例中,我们使用exit() 函数在 'i' 达到 5 时立即终止进程。
#include <stdio.h> #include <stdlib.h> int main(){ // print number from 0 to 5 int i; for(i=0;i<=7;i++){ // if i is equals to 5 then terminates if(i==5){ exit(0); } else{ printf("%d\n",i); } } return 0; }
输出
以下是输出 -
0 1 2 3 4
示例 3
这是一个获取用户详细信息的另一个程序。如果用户详细信息与 if 条件匹配,则 'exit(EXIT_SUCCESS)' 将起作用。否则 'exit(EXIT_FAILURE)'。
#include <stdio.h> #include <stdlib.h> #include <string.h> int main() { const char *username = "tutorialspoint"; const char *password = "tp1234"; // Check the credentials if (strcmp(username, "tutorialspoint") == 0 && strcmp(password, "tp1234") == 0){ printf("Login successful!\n"); // if user details match exit exit(EXIT_SUCCESS); } else { fprintf(stderr, "Invalid credentials!\n"); // if user details not match exit exit(EXIT_FAILURE); } return 0; }
输出
以下是输出 -
Login successful!
广告