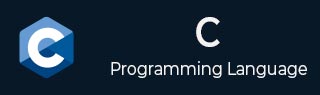
- C标准库
- C库 - 首页
- C库 - <assert.h>
- C库 - <complex.h>
- C库 - <ctype.h>
- C库 - <errno.h>
- C库 - <fenv.h>
- C库 - <float.h>
- C库 - <inttypes.h>
- C库 - <iso646.h>
- C库 - <limits.h>
- C库 - <locale.h>
- C库 - <math.h>
- C库 - <setjmp.h>
- C库 - <signal.h>
- C库 - <stdalign.h>
- C库 - <stdarg.h>
- C库 - <stdbool.h>
- C库 - <stddef.h>
- C库 - <stdio.h>
- C库 - <stdlib.h>
- C库 - <string.h>
- C库 - <tgmath.h>
- C库 - <time.h>
- C库 - <wctype.h>
C库 - fegetexceptflag() 函数
C 的fenv库 fegetexceptflag() 函数用于检索由'excepts'参数指定的浮点异常标志的当前状态或完整内容。此参数是浮点异常宏的按位 OR 组合,例如FE_DIVBYZERO, FE_INEXACT, FE_INVALID, FE_OVERFLOW, 和 FE_UNDERFLOW。
浮点异常标志的完整内容不仅仅是指示是否发生异常的真/假值。相反,它可能更复杂,例如一个结构体,其中包含异常的状态以及其他详细信息,例如导致异常的代码的地址。
语法
以下是fegetexceptflag() 函数的C库语法:
int fegetexceptflag( fexcept_t* flagp, int excepts );
参数
此函数接受以下参数:
-
flagp − 它表示指向'fexcept_t'对象的指针,标志将存储在其中或从中读取。
excepts − 它表示要获取的异常标志的位掩码列表。
返回值
如果成功获取指定异常的状态,则此函数返回 0;否则返回非零值。
示例 1
以下是演示fegetexceptflag() 函数用法的基本C程序。
#include <stdio.h> #include <fenv.h> int main() { // Declare a variable to hold the exception flags fexcept_t flag; // Perform some floating-point operations that may raise exceptions double x = 1.0 / 0.0; // retrieve the state of the FE_DIVBYZERO exception flag if (fegetexceptflag(&flag, FE_DIVBYZERO) != 0) { printf("Failed to obtain the state of the FE_DIVBYZERO exception flag.\n"); return 1; } else { printf("Successfully obtained the state of the FE_DIVBYZERO exception flag.\n"); } return 0; }
输出
以下是输出:
Successfully obtained the state of the FE_DIVBYZERO exception flag.
示例 2
以下C程序使用fegetexceptflag() 函数。在这里,我们获取多个异常标志。
#include <stdio.h> #include <fenv.h> int main() { fexcept_t flag; // Perform some floating-point operations that may raise exceptions double x = 0.0 / 0.0; double y = 1e308 * 1e308; // Get the state of the FE_INVALID and FE_OVERFLOW exception flags if (fegetexceptflag(&flag, FE_INVALID | FE_OVERFLOW) != 0) { printf("Failed to obtain the state of the FE_INVALID and FE_OVERFLOW exception flags.\n"); return 1; } else { printf("Successfully obtained the state of the FE_INVALID and FE_OVERFLOW exception flags.\n"); } return 0; }
输出
以下是输出:
Successfully obtained the state of the FE_INVALID and FE_OVERFLOW exception flags.
示例 3
下面的示例,如果成功获取指定异常的状态,则返回 0。
#include <stdio.h> #include <fenv.h> #include <math.h> int main() { fexcept_t flag; // Perform some floating-point operations that may raise exceptions double x = 10.0 / 0.0; double square_root = sqrt(-1.0); // Get the state of the FE_INVALID and FE_OVERFLOW exception flags int res = fegetexceptflag(&flag, FE_INVALID | FE_OVERFLOW); printf("%d", res); return 0; }
输出
以下是输出:
0
c_library_fenv_h.htm
广告