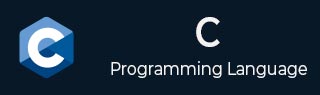
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - fmod() 函数
C 库的 fmod() 函数接受两个类型为 double 的参数 (x & y),返回除法 (x/y) 的余数。
简单来说,它计算两个数字相除的浮点余数。
语法
以下是 C 库函数 fmod() 的语法 -
double fmod(double x, double y)
参数
此函数仅接受两个参数 -
x - 这是作为除法分子使用的浮点数。
y - 这是作为除法分母使用的浮点数。
返回值
此函数返回 x/y 相除的余数。
示例 1
C 库程序说明了 fmod() 函数的使用方法。
#include <stdio.h> #include <math.h> int main() { float p = fmod(13, -7); float q = fmod(9, 3); float r = fmod(7, 10); float s = fmod(5, 2); printf("%f %f %f %f", p, q, r, s); return 0; }
输出
执行上述代码后,我们得到以下结果 -
6.000000 0.000000 7.000000 1.000000
示例 2
这里,我们使用了两个浮点值和一个整数,演示了使用 fmod() 计算余数的操作。
#include <stdio.h> #include <math.h> int main () { float a, b; int c; a = 9.2; b = 3.7; c = 2; printf("Remainder of %f / %d is %lf\n", a, c, fmod(a,c)); printf("Remainder of %f / %f is %lf\n", a, b, fmod(a,b)); return(0); }
输出
上述代码产生以下结果 -
Remainder of 9.200000 / 2 is 1.200000 Remainder of 9.200000 / 3.700000 is 1.800000
示例 3
给定的程序有两个浮点值 (x,y),将其传递给 fmod() 函数以计算其余数并显示结果。
#include <stdio.h> #include <math.h> int main() { double x = 10.5, y = 6.5; double result = fmod(x, y); printf("Remainder of %f / %f == %f\n", x, y, result); return 0; }
输出
执行代码后,我们得到以下结果 -
Remainder of 10.500000 / 6.500000 == 4.000000
广告