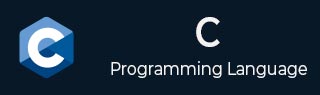
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - fsetpos() 函数
C 库的 fsetpos() 函数将给定流的文件位置设置为给定位置。参数 pos 是由 fgetpos 函数给出的位置。此函数是 <stdio.h> 库的一部分,对于文件的随机访问特别有用。
语法
以下是 C 库 fsetpos() 函数的语法:
int fsetpos(FILE *stream, const fpos_t *pos);
参数
此函数接受以下参数:
- FILE *stream: 指向 FILE 对象的指针,标识该流。
- const fpos_t *pos: 指向 fpos_t 对象的指针,包含新的位置。fpos_t 类型用于表示文件位置。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
返回值
fsetpos() 函数成功返回 0,出错返回非零值。
示例 1
此示例演示在写入文件后将文件位置设置为文件开头。
#include <stdio.h> int main() { FILE *file = fopen("example1.txt", "w+"); if (file == NULL) { perror("Failed to open file"); return 1; } fputs("Hello, World!", file); fpos_t pos; fgetpos(file, &pos); // Get the current position rewind(file); // Set position to the beginning fsetpos(file, &pos); // Set back to the saved position fputs(" Overwrite", file); fclose(file); return 0; }
输出
上述代码产生以下结果:
Hello, World! Overwrite
示例 2
使用 fsetpos() 移动到特定位置
此示例演示如何移动到文件中的特定位置并修改内容。
#include <stdio.h> int main() { FILE *file = fopen("example2.txt", "w+"); if (file == NULL) { perror("Failed to open file"); return 1; } fputs("1234567890", file); fpos_t pos; fgetpos(file, &pos); // Get the current position pos.__pos = 5; // Set position to 5 fsetpos(file, &pos); // Move to position 5 fputs("ABCDE", file); fclose(file); return 0; }
输出
执行上述代码后,我们将得到以下结果:
12345ABCDE
示例 3
保存和恢复文件位置
此示例演示如何保存文件位置,执行某些操作,然后恢复原始位置。
#include <stdio.h> int main() { FILE *file = fopen("example3.txt", "w+"); if (file == NULL) { perror("Failed to open file"); return 1; } fputs("Original content", file); fpos_t pos; fgetpos(file, &pos); // Save the current position fseek(file, 0, SEEK_END); // Move to the end of the file fputs("\nNew content", file); fsetpos(file, &pos); // Restore the original position fputs(" modified", file); fclose(file); return 0; }
输出
上述代码的输出如下:
Original modified content New content
广告