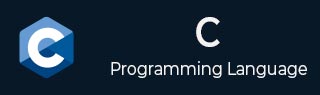
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - getc() 函数
C 库函数 getc(FILE *stream) 从指定的流中获取下一个字符(一个无符号字符),并推进流的位置指示器。它对于在字符级别处理文件输入操作至关重要。
语法
以下是 C 库函数 getc() 的语法:
int getc(FILE *stream);
参数
此函数仅接受一个参数:
- FILE *stream: 指向 FILE 对象的指针,指定输入流。此 FILE 对象通常使用 fopen 函数获取。
返回值
成功时,函数返回从指定流中获取的下一个字符,作为转换为 int 类型的无符号 char;失败时,如果发生错误或到达文件结尾,getc 返回 EOF(在 <stdio.h> 中定义的常量)。
示例 1:从文件读取字符
此示例演示如何使用 getc 从文件读取字符并将其打印到标准输出。
以下是 C 库 getc() 函数的示例。
#include <stdio.h> int main() { FILE *file = fopen("example1.txt", "r"); if (file == NULL) { perror("Error opening file"); return 1; } int ch; while ((ch = getc(file)) != EOF) { putchar(ch); } fclose(file); return 0; }
输出
以上代码读取 example1.txt 文件的内容,然后产生以下结果:
Hello, World!
示例 2:读取直到特定字符
此示例从文件中读取字符,直到遇到字符“!”,演示了使用 getc 进行条件读取。
#include <stdio.h> int main() { FILE *file = fopen("example3.txt", "r"); if (file == NULL) { perror("Error opening file"); return 1; } int ch; while ((ch = getc(file)) != EOF && ch != '!') { putchar(ch); } fclose(file); return 0; }
输出
假设 example3.txt 文件包含文本:“Stop reading here! Continue...” ,则以上代码产生以下结果:
Stop reading here
广告