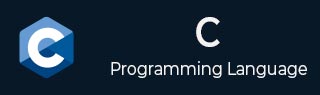
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - isspace() 函数
C 库的 isspace() 函数检查传递的字符是否为空格字符。
此函数是 C 标准库的一部分,包含在 <ctype.h> 头文件中。
标准空格字符包括:
' ' (0x20) space (SPC) '\t' (0x09) horizontal tab (TAB) '\n' (0x0a) newline (LF) '\v' (0x0b) vertical tab (VT) '\f' (0x0c) feed (FF) '\r' (0x0d) carriage return (CR)
语法
以下是 C 库 isspace() 函数的语法:
int isspace(int c);
参数
此函数接受单个参数:
c − 要检查的字符,作为 int 类型传递。c 的值应可表示为无符号字符或等于 EOF 的值。
返回值
如果字符是空格字符,则 isspace(int c) 函数返回非零值 (true)。否则,它返回零 (false)。isspace 检查的空格字符包括:
- 空格 (' ')
- 换页符 ('\f')
- 换行符 ('\n')
- 回车符 ('\r')
- 水平制表符 ('\t')
- 垂直制表符 ('\v')
示例 1:检查不同的输入是否为空格
使用 isspace() 函数检查字符 't'、数字 '1' 和空格 '' 是否被识别为空格。
#include <stdio.h> #include <ctype.h> int main () { int var1 = 't'; int var2 = '1'; int var3 = ' '; if( isspace(var1) ) { printf("var1 = |%c| is a white-space character\n", var1 ); } else { printf("var1 = |%c| is not a white-space character\n", var1 ); } if( isspace(var2) ) { printf("var2 = |%c| is a white-space character\n", var2 ); } else { printf("var2 = |%c| is not a white-space character\n", var2 ); } if( isspace(var3) ) { printf("var3 = |%c| is a white-space character\n", var3 ); } else { printf("var3 = |%c| is not a white-space character\n", var3 ); } return(0); }
输出
以上代码产生以下结果:
var1 = |t| is not a white-space character var2 = |1| is not a white-space character var3 = | | is a white-space character
示例 2:换行符
此示例检查换行符 ('\n') 是否为空格字符。由于它是空格字符,因此函数返回 true。
#include <stdio.h> #include <ctype.h> int main() { char ch = '\n'; if (isspace(ch)) { printf("The character is a whitespace character.\n"); } else { printf("The character is not a whitespace character.\n"); } return 0; }
输出
执行以上代码后,我们得到以下结果:
The character is a whitespace character.
广告