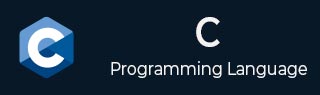
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - iswalpha() 函数
C 的wctype 库 iswalpha() 函数用于检查给定的宽字符(由 wint_t 表示)是否为字母字符,即大写字母、小写字母或当前区域设置特有的任何字母字符。
此函数可用于字符验证、大小写转换、密码验证、字符串处理或标记化和解析。
语法
以下是 iswalpha() 函数的 C 库语法:
int iswalpha( wint_t ch )
参数
此函数接受一个参数:
-
ch - 它是要检查的类型为 'wint_t' 的宽字符。
返回值
如果宽字符是字母字符,则此函数返回非零值,否则返回零。
示例 1
以下是演示 iswalpha() 函数用法的基本 C 示例。
#include <wctype.h> #include <stdio.h> int main() { wint_t ch = L'T'; if (iswalpha(ch)) { printf("The wide character %lc is alphabetic.\n", ch); } else { printf("The wide character %lc is not alphabetic.\n", ch); } return 0; }
输出
以下是输出:
The wide character T is alphabetic.
示例 2
在这里,我们使用 iswalpha() 函数打印字母字符。
#include <stdio.h> #include <wctype.h> #include <wchar.h> int main() { wchar_t wc[] = L"tutorialspoint 500081"; // Iterate over each character in the wide string for (int i = 0; wc[i] != L'\0'; i++) { if (iswalpha(wc[i])) { wprintf(L"%lc", wc[i]); } } return 0; }
输出
以下是输出:
tutorialspoint
示例 3
让我们创建一个另一个 C 程序来提取并打印宽字符中的字母字符。
#include <stdio.h> #include <wctype.h> #include <wchar.h> int main() { wchar_t wc[] = L"2006 tutorialspoint India Pvt.Ltd 2024"; wchar_t alphabetic[100]; int index = 0; // Iterate over each character in the wide string for (int i = 0; wc[i] != L'\0'; i++) { if (iswalpha(wc[i])) { alphabetic[index++] = wc[i]; } } // null terminated string alphabetic[index] = L'\0'; // print the alphabetic character wprintf(L"The alphabetic characters in the wide string \"%ls\" \n are \"%ls\".\n", wc, alphabetic); return 0; }
输出
以下是输出:
The alphabetic characters in the wide string "2006 tutorialspoint India Pvt.Ltd 2024" are "tutorialspointIndiaPvtLtd".
c_library_wctype_h.htm
广告