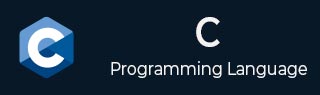
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - iswblank() 函数
C 的wctype 库 iswblank() 函数用于检查给定的宽字符(类型为 wint_t)是否被归类为空白字符。
空白包括当前 C 语言区域设置中分隔句子中单词的空格。在默认的 C 语言区域设置中,只有空格 (0x20) 和水平制表符 (0x09) 是空白字符。
此函数可用于文本处理程序、用户输入验证、文字处理和文本编辑器工具等。
语法
以下是 iswblank() 函数的 C 库语法 -
int iswblank( wint_t ch );
参数
此函数接受一个参数 -
-
ch - 它是要检查的类型为 'wint_t' 的宽字符。
返回值
如果宽字符是空白字符,则此函数返回非零值,否则返回零。
示例 1
以下是演示 iswblank() 用法的基本 c 示例。
#include <locale.h> #include <stdio.h> #include <wchar.h> #include <wctype.h> int main(void) { // Set the locale to "en_US.utf8" setlocale(LC_ALL, "en_US.utf8"); wchar_t wchar = L'\t'; if(iswblank(wchar)==1){ printf("Character '%lc' (%#x) is blank character\n", wchar, wchar); } else{ printf("Character '%lc' (%#x) is not a blank character\n", wchar, wchar); } return 0; }
输出
以下是输出 -
Character ' ' (0x9) is blank character
示例 2
让我们创建另一个 c 程序,并使用 iswblank() 来验证数组的元素是否为空白。
#include <locale.h> #include <stdio.h> #include <wchar.h> #include <wctype.h> int main(void) { // Set the locale to "en_US.utf8" setlocale(LC_ALL, "en_US.utf8"); // Define an array of wide characters wchar_t wchar[] = { L'A', L' ', L'\t', L'@' }; size_t len = sizeof(wchar) / sizeof(wchar[0]); printf("Checking blank characters:\n"); for (size_t i = 0; i < len; ++i) { wchar_t ch = wchar[i]; printf("Character '%lc' (%#x) is %s blank character\n", ch, ch, iswblank(ch) ? "a" : "not a"); } return 0; }
输出
以下是输出 -
Checking blank characters: Character 'A' (0x41) is not a blank character Character ' ' (0x20) is a blank character Character ' ' (0x9) is a blank character Character '@' (0x40) is not a blank character
示例 3
下面的 c 示例使用 iswblank() 函数在遇到空白后中断语句。
#include <stdio.h> #include <wchar.h> #include <wctype.h> int main(void) { // wide char array wchar_t wc[] = L"Tutrialspoint India pvt ltd"; // find the length of wide char size_t len = wcslen(wc); for (size_t i = 0; i < len; ++i) { // Check if the current character is blank if (iswblank(wc[i])) { // Print a newline character if blanck is found putchar('\n'); } else { // Print the current character if it's not blank putchar(wc[i]); } } return 0; }
输出
以下是输出 -
Tutrialspoint India pvt ltd
c_library_wctype_h.htm
广告