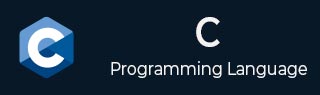
- C标准库
- C库 - 首页
- C库 - <assert.h>
- C库 - <complex.h>
- C库 - <ctype.h>
- C库 - <errno.h>
- C库 - <fenv.h>
- C库 - <float.h>
- C库 - <inttypes.h>
- C库 - <iso646.h>
- C库 - <limits.h>
- C库 - <locale.h>
- C库 - <math.h>
- C库 - <setjmp.h>
- C库 - <signal.h>
- C库 - <stdalign.h>
- C库 - <stdarg.h>
- C库 - <stdbool.h>
- C库 - <stddef.h>
- C库 - <stdio.h>
- C库 - <stdlib.h>
- C库 - <string.h>
- C库 - <tgmath.h>
- C库 - <time.h>
- C库 - <wctype.h>
C库 - malloc() 函数
C 的stdlib库 malloc() 函数用于动态内存分配。它分配或预留指定字节数的内存块,并返回指向已分配空间第一个字节的指针。
malloc() 函数在编译时不知道所需内存大小,而必须在运行时确定时使用。
语法
以下是 malloc() 函数的C库语法:
void *malloc(size_t size)
参数
此函数接受单个参数:
size_t size − 表示要分配的字节数。
返回值
以下是返回值:
返回指向新分配的内存块开头的指针。
如果分配失败,则返回空指针。
示例1
让我们创建一个基本的C程序来演示 malloc() 函数的使用。
#include <stdio.h> #include <stdlib.h> int main() { int *pointer = (int *)calloc(0, 0); if (pointer == NULL) { printf("Null pointer \n"); } else { printf("Address = %p", (void *)pointer); } free(pointer); return 0; }
输出
以下是输出:
Address = 0x55c6799232a0
示例2
以下是另一个 malloc() 的示例。这里它用于为包含5个整数的数组分配内存。
#include <stdio.h> #include <stdlib.h> int main() { int *ptr; int n = 5; // Dynamically allocate memory using malloc() ptr = (int*)malloc(n * sizeof(int)); // Check if the memory has been successfully allocated if (ptr == NULL) { printf("Memory not allocated.\n"); exit(0); } else { // Memory has been successfully allocated printf("Memory successfully allocated using malloc.\n"); // Free the memory free(ptr); printf("Malloc memory successfully freed.\n"); } return 0; }
输出
以下是输出:
Memory successfully allocated using malloc. Malloc memory successfully freed.
示例3
下面的C程序将数组元素动态存储在指针中,并计算数组元素的总和。
#include <stdio.h> #include <stdlib.h> int main() { int n = 5, i, *ptr, sum = 0; int arr[] = {10, 20, 30, 40, 50}; ptr = (int*) malloc(n * sizeof(int)); // if memory cannot be allocated if(ptr == NULL) { printf("Error! memory not allocated."); exit(0); } // Copy values from arr to dynamically allocated memory for(i = 0; i < n; ++i) { *(ptr + i) = arr[i]; sum += *(ptr + i); } printf("Sum = %d", sum); // free the allocated memory free(ptr); return 0; }
输出
以下是输出:
Sum = 150
广告