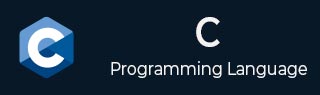
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - mbtowc() 函数
C 的stdlib库的mbtowc()函数用于将'str'指向的多字节字符字符串转换为其宽字符表示形式。此函数与“mbstowcs”相同。
转换后的字符存储在'pwc'指向的数组的连续元素中。
目标数组最多只能容纳 n 个宽字符。
语法
以下是mbtowc()函数的 C 库语法 -
int mbtowc(whcar_t *pwc, const char *str, size_t n)
参数
此函数接受以下参数 -
pwc - 它表示指向 wchar_t 元素数组的指针,该数组足够长,可以存储最多 n 个字符的宽字符串。
str - 它表示指向需要转换的空终止多字节字符串的第一个元素的指针。
n - 它表示'pwc'指向的数组中存在的最大 wchar_t 字符数。
返回值
此函数返回转换的字符数,不包括空字符。如果找到无效的多字节字符,则返回 -1。
示例 1
在此示例中,我们创建了一个基本的 c 程序来演示mbtowc()函数的使用。
#include <stdio.h> #include <stdlib.h> #include <wchar.h> void mbtowc_func(const char *ptr, size_t max) { int length; wchar_t dest_arr; // Reset mbtowc internal state mbtowc(NULL, NULL, 0); while (max > 0) { length = mbtowc(&dest_arr, ptr, max); if (length < 1) { break; } // Print each character in square braces printf("[%lc]", dest_arr); ptr += length; max -= length; } } int main() { const char str[] = "tutorialspoint India"; mbtowc_func(str, sizeof(str)); return 0; }
输出
以下是输出 -
[t][u][t][o][r][i][a][l][s][p][o][i][n][t][ ][I][n][d][i][a]
示例 2
以下 c 示例使用mbtowc()函数将多字节字符串转换为宽字符字符串。
#include <stdio.h> #include <stdlib.h> #include <locale.h> #include <wchar.h> int main() { // Set the locale to handle multibyte characters setlocale(LC_ALL, ""); const char mbString[] = "This is tutorialspoint.com"; wchar_t wideChar; size_t i; for (i = 0; i < sizeof(mbString);) { int length = mbtowc(&wideChar, &mbString[i], MB_CUR_MAX); if (length > 0) { // Print each wide character in square braces wprintf(L"[%lc]", wideChar); i += length; } else { break; } } return 0; }
输出
以下是输出 -
[T][h][i][s][ ][i][s][ ][t][u][t][o][r][i][a][l][s][p][o][i][n][t][.][c][o][m]
示例 3
让我们再创建一个示例,使用mbtowc()函数将无效的多字节序列转换为宽字符。
#include <stdio.h> #include <stdlib.h> #include <wchar.h> #include <locale.h> int main() { // Set the locale to the user's default locale setlocale(LC_ALL, ""); // An invalid multibyte sequence const char *invalid_mb_str = "\xC3\x28"; wchar_t ptr; int len; len = mbtowc(&ptr, invalid_mb_str, MB_CUR_MAX); if (len == -1) { printf("Invalid multibyte sequence.\n"); } else { printf("Converted wide character: %lc\n", ptr); } return 0; }
输出
以下是输出 -
Invalid multibyte sequence.
广告