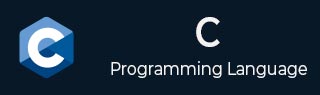
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - pow() 函数
C 库的 pow() 函数是 double 类型,它接受参数 x 和 y,计算 x 的 y 次幂。
此函数允许程序员计算底数的指定次幂,而无需手动实现复杂的乘法循环。
语法
以下是 C 库函数 pow() 的语法:
double pow(double x, double y)
参数
此函数仅接受两个参数:
x - 这是浮点型底数。
y - 这是浮点型指数。
返回值
此函数返回 x 的 y 次幂的结果。
示例 1
以下是一个基本的 C 程序,演示了 pow() 函数的用法。
#include <stdio.h> #include <math.h> int main () { printf("Value 8.0 ^ 3 = %lf\n", pow(8.0, 3)); printf("Value 3.05 ^ 1.98 = %lf", pow(3.05, 1.98)); return(0); }
输出
以上代码的输出结果如下:
Value 8.0 ^ 3 = 512.000000 Value 3.05 ^ 1.98 = 9.097324
示例 2
在下面的程序中,我们使用 pow() 函数演示了给定底数的幂表。
#include <stdio.h> #include <math.h> int main() { double base; printf("Enter the base number: "); scanf("%lf", &base); printf("Powers of %.2lf:\n", base); for (int i = 0; i <= 10; ++i) { double result = pow(base, i); printf("%.2lf ^ %d = %.2lf\n", base, i, result); } return 0; }
输出
执行以上代码后,得到以下结果:
Enter the base number: 5 Powers of 5.00: 5.00 ^ 0 = 1.00 5.00 ^ 1 = 5.00 5.00 ^ 2 = 25.00 5.00 ^ 3 = 125.00 5.00 ^ 4 = 625.00 5.00 ^ 5 = 3125.00 5.00 ^ 6 = 15625.00 5.00 ^ 7 = 78125.00 5.00 ^ 8 = 390625.00 5.00 ^ 9 = 1953125.00 5.00 ^ 10 = 9765625.00
示例 3
在这里,我们创建一个程序,接受用户输入的底数和指数,使用 pow() 函数计算结果,并显示结果。
#include <stdio.h> #include <math.h> int main() { double base, exponent, result; printf("Enter the base number: "); scanf("%lf", &base); printf("Enter the exponent: "); scanf("%lf", &exponent); result = pow(base, exponent); printf("%.2lf ^ %.2lf = %.2lf\n", base, exponent, result); return 0; }
输出
执行代码后,得到以下结果:
Enter the base number: 5 Enter the exponent: 2 5.00 ^ 2.00 = 25.00 Or, Enter the base number: 3 Enter the exponent: 3 3.00 ^ 3.00 = 27.00
广告