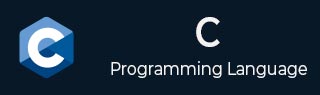
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - qsort() 函数
C 的stdlib 库 qsort() 函数是一个排序函数,用于对数组进行升序或降序排序。它被称为快速排序。
此函数基于快速排序算法,该算法是最快速和最高效的排序算法之一。
语法
以下是 qsort() 函数的 C 库语法:
void qsort(void *base, size_t nitems, size_t size, int (*compar)(const void *, const void*))
参数
此函数接受以下参数:
base - 表示指向要排序的数组第一个元素的指针。
nitems - 表示数组中的元素个数。
size - 表示数组中每个元素的大小。
compare - 表示指向比较函数的函数指针,该函数比较两个元素。
返回值
此函数不返回任何值。
示例 1
在这个示例中,我们创建一个基本的 C 程序来演示 qsort() 函数的使用。
#include <stdio.h> #include <stdlib.h> // Comparison function int compare(const void* a, const void* b) { return (*(int*)a - *(int*)b); } int main() { int arr[] = {10, 5, 4, 6, 9}; int n = sizeof(arr) / sizeof(arr[0]); qsort(arr, n, sizeof(int), compare); printf("Following is the sorted array: "); int i; for (i = 0; i < n; ++i) { printf("%d ", arr[i]); } printf("\n"); return 0; }
以下是输出:
Following is the sorted array: 4 5 6 9 10
示例 2
下面的 C 程序对数组的字符进行排序。使用 qsort() 函数。
#include <string.h> #include <stdio.h> #include <stdlib.h> // Comparison function int compare(const void *arg1, const void *arg2){ return strcasecmp(*(const char **)arg1, *(const char **)arg2); } int main(void){ char *colors[] = {"B", "D", "A", "W", "Z", "X", "M", "O"}; int i; // Size of the array int size = sizeof(colors) / sizeof(colors[0]); printf("Original array elements:\n"); for (i = 0; i < size; i++) { printf("%s ", colors[i]); } printf("\n\n"); // Use qsort to sort qsort(colors, size, sizeof(char *), compare); printf("Following is the sorted array: "); for (i = 0; i < size; ++i) { printf("%s ", colors[i]); } return 0; }
输出
以下是输出:
Original array elements: B D A W Z X M O Following is the sorted array: A B D M O W X Z
示例 3
让我们再创建一个 C 程序来对数组的字符串进行排序。使用 qsort() 函数。
#include <string.h> #include <stdio.h> #include <stdlib.h> // Comparison function int compare(const void *arg1, const void *arg2){ return strcasecmp(*(const char **)arg1, *(const char **)arg2); } int main(void){ char *colors[] = {"Vivek", "Aman", "Shriansh", "Tapas"}; int i; // Size of the array int size = sizeof(colors) / sizeof(colors[0]); printf("Original array elements:\n"); for (i = 0; i < size; i++) { printf("%s ", colors[i]); } printf("\n"); // Use qsort to sort qsort(colors, size, sizeof(char *), compare); printf("Following is the sorted array: "); for (i = 0; i < size; ++i) { printf("%s ", colors[i]); } return 0; }
输出
以下是输出:
Original array elements: Vivek Aman Shriansh Tapas Following is the sorted array: Aman Shriansh Tapas Vivek
广告