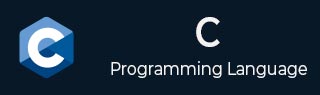
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - rand() 函数
C 的stdlib 库的rand() 函数用于返回范围在 0 到 RAND_MAX 之间的伪随机数。
RAND_MAX 表示 rand() 函数可以返回的最大值,其默认值可能因 C 库的实现而异。但它保证至少为 32767。
语法
以下是 rand() 函数的 C 库语法:
int rand(void)
参数
此函数不接受任何参数。
返回值
此函数返回一个介于 0 到 RAND_MAX 之间的整数值。
示例 1
在本例中,我们创建一个基本的 C 程序来演示 rand() 函数的使用。
#include <stdio.h> #include <stdlib.h> int main() { // store the random value int rand_value = rand(); // display the random value printf("Random Value: %d\n", rand_value); return 0; }
以下是输出:
Random Value: 1804289383
示例 2
下面的 C 程序用于使用 rand() 函数在一定范围内显示随机数。
#include <stdio.h> #include <stdlib.h> int main() { // display the random value int i; for(i=1; i<=5; i++) { printf("%d ", rand()); } return 0; }
输出
以下是输出:
1804289383 846930886 1681692777 1714636915 1957747793
示例 3
让我们再创建一个示例,我们正在创建一个 C 程序来生成将是 10 的倍数的随机数。
#include <stdio.h> #include <stdlib.h> int main() { // display the random value int num = 10; int i; for(i=1; i<=6; i++) { printf("%d ", rand()%num); } return 0; }
输出
以下是输出:
3 6 7 5 3 5
广告