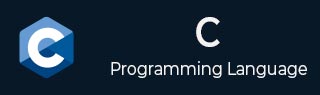
- C标准库
- C库 - 首页
- C库 - <assert.h>
- C库 - <complex.h>
- C库 - <ctype.h>
- C库 - <errno.h>
- C库 - <fenv.h>
- C库 - <float.h>
- C库 - <inttypes.h>
- C库 - <iso646.h>
- C库 - <limits.h>
- C库 - <locale.h>
- C库 - <math.h>
- C库 - <setjmp.h>
- C库 - <signal.h>
- C库 - <stdalign.h>
- C库 - <stdarg.h>
- C库 - <stdbool.h>
- C库 - <stddef.h>
- C库 - <stdio.h>
- C库 - <stdlib.h>
- C库 - <string.h>
- C库 - <tgmath.h>
- C库 - <time.h>
- C库 - <wctype.h>
C库 - realloc() 函数
C 的stdlib 库 realloc() 函数用于重新分配动态分配内存的大小。它允许我们在释放先前分配的内存后分配或预留一个新的内存块。
realloc() 函数用于优化内存使用。如果我们发现分配的内存太大或太小,我们可以使用 realloc() 来调整分配内存的大小。
此函数仅在使用 realloc() 函数之前已动态分配内存时才有效。
语法
以下是 realloc() 函数的 C 库语法:
void *realloc(void *ptr, size_t size)
参数
此函数接受以下参数:
-
ptr - 它表示要重新分配的内存块的指针。该指针先前由 'malloc()'、'calloc()' 和 'realloc()' 分配。
-
size - 它表示内存块的新大小(以字节为单位)。如果大小为零,且指针非空,则会分配一个新的最小大小的对象,原始对象将被释放。
返回值
此函数返回指向新分配内存的指针。如果函数未能分配请求的内存块,则返回 'NULL'。
示例 1
在此示例中,我们在没有动态分配内存的情况下使用 realloc() 函数。
#include <stdio.h> #include <stdlib.h> int main() { // initialize an array of size 10 int arr[10]; // store the array to pointer int *ptr = arr; // new pointer for reallocating int *new_ptr; arr[0] = 5; arr[1] = 10; // use the new pointer new_ptr = (int *)realloc(ptr, sizeof(int)*5); *(new_ptr + 2) = 30; int i; for(i = 0; i < 5; i++){ printf("%d ", *(new_ptr + i)); } // use getchar to get the charcter // if memory is allocated getchar(); return 0; }
输出
以下是我们在没有动态分配内存的情况下使用 realloc() 时的输出。
warning: 'realloc' called on unallocated object 'arr' [-Wfree-nonheap-object] new_ptr = (int *)realloc(ptr, sizeof(int)*5); ^~~~~~~~~~~~~~~~~~~~~~~~~ note: declared here int arr[10]; Segmentation fault
示例 2
下面的示例使用 realloc() 来增加内存块的大小。
#include <stdio.h> #include <stdlib.h> int main() { // Initially allocate memory for 2 integers int *ptr = malloc(2 * sizeof(int)); if (ptr == NULL) { fprintf(stderr, "Memory allocation failed\n"); return 1; } ptr[0] = 5; ptr[1] = 10; // increase the size of the integer int *ptr_new = realloc(ptr, 3 * sizeof(int)); if (ptr_new == NULL) { fprintf(stderr, "Memory reallocation failed\n"); free(ptr); return 1; } ptr_new[2] = 15; int i; // Display the array for (i = 0; i < 3; i++) { printf("%d ", ptr_new[i]); } free(ptr_new); return 0; }
输出
以下是输出:
5 10 15
示例 3
让我们创建另一个示例,我们先将数组元素动态存储到指针中,然后将数组大小增加 7。
#include <stdio.h> #include <stdlib.h> int main() { int *ptr, i; printf("Initial size of the array is 4\n"); // Allocate memory for 4 integers ptr = (int*)calloc(4, sizeof(int)); if(ptr == NULL) { printf("Memory allocation failed"); exit(1); } // initial array int initialArray[4] = {1, 2, 3, 4}; // Copy array into ptr for(i = 0; i < 4; i++) { ptr[i] = initialArray[i]; } printf("Increasing the size of the array by 3 elements ...\n"); // Increase the size of the array to 7 elements // use realloc ptr = (int*)realloc(ptr, 7 * sizeof(int)); if(ptr == NULL) { printf("Memory allocation failed"); exit(1); } // additional elements int additionalElements[3] = {5, 6, 7}; // Copy additional elements into ptr for(i = 4; i < 7; i++) { ptr[i] = additionalElements[i - 4]; } printf("Final array: \n"); // display the final array for(i = 0; i < 7; i++) { printf("%d ", *(ptr+i)); } free(ptr); return 0; }
输出
以下是输出:
Initial size of the array is 4 Increasing the size of the array by 3 elements ... Final array: 1 2 3 4 5 6 7
广告