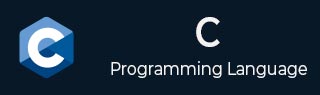
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - setlocale() 函数
C 库的 setlocale() 函数设置或读取与位置相关的 信息。此函数在特定系统中安装系统区域设置。为了修改剩余的影响,它在下次调用 setlocale 之前执行所有 C STL 的敏感函数。
语法
以下是 C setlocale() 函数的语法:
char *setlocale(int category, const char *locale)
参数
以下是不同区域设置类别的列表:
category - 这是一个命名常量,指定受区域设置影响的函数类别。
- LC_ALL 用于以下所有类别。
- LC_COLLATE 用于字符串比较。参见 strcoll()。
- LC_CTYPE 用于字符分类和转换。例如:strtoupper()。
- LC_MONETARY 用于货币格式化,用于 localeconv()。
- LC_NUMERIC 用于十进制分隔符,用于 localeconv()。
- LC_TIME 用于使用 strftime() 进行日期和时间格式化。
- LC_MESSAGES 用于系统响应。
- locale - 如果 locale 为 NULL 或空字符串 (""),则区域设置名称将从与上述类别名称相同的环境变量的值中设置。
返回值
setlocale() 函数调用成功则返回一个与已设置区域设置对应的、不透明的字符串。如果请求无法满足,则返回值为 NULL。
示例 1
以下是一个基本的 C 程序,用于演示 setlocale() 函数的用法。
#include <locale.h> #include <stdio.h> #include <time.h> int main () { time_t currtime; struct tm *timer; char buffer[80]; time( &currtime ); timer = localtime( &currtime ); printf("Locale is: %s\n", setlocale(LC_ALL, "en_GB")); strftime(buffer,80,"%c", timer ); printf("Date is: %s\n", buffer); printf("Locale is: %s\n", setlocale(LC_ALL, "de_DE")); strftime(buffer,80,"%c", timer ); printf("Date is: %s\n", buffer); return(0); }
输出
执行代码后,我们将得到以下结果:
Locale is: en_GB Date is: Fri 05 Dec 2014 10:35:02 UTC Locale is: de_DE Date is: Fr 05 Dez 2014 10:35:02 UTC
示例 2
在此示例中,我们通过使用 setlocale() 函数来说明使用 LC_Numeric 区域设置的十进制分隔符。
#include <stdio.h> #include <locale.h> int main() { // Set the locale to the user's default locale setlocale(LC_NUMERIC, ""); // Get the current locale for LC_NUMERIC char *currentLocale = setlocale(LC_NUMERIC, NULL); printf("Current LC_NUMERIC locale: %s\n", currentLocale); // Display the floating-point number with the current locale double number = 65743.6789; printf("Formatted number: %.2f\n", number); return 0; }
输出
执行上述代码后,我们将得到以下结果:
Current LC_NUMERIC locale: en_US.UTF-8 Formatted number: 65743.68
示例 3
在此示例中,我们使用 setlocale() 函数来设置 LC_TIME(本地化),以显示日期和时间格式。
#include <stdio.h> #include <time.h> #include <locale.h> int main() { // Set the locale to the user's default locale setlocale(LC_TIME, ""); // Get the current locale for LC_TIME char *currentLocale = setlocale(LC_TIME, NULL); printf("Current LC_TIME locale: %s\n", currentLocale); // Display the current date and time time_t currentTime; struct tm *timeInfo; time(¤tTime); timeInfo = localtime(¤tTime); char formattedTime[100]; strftime(formattedTime, sizeof(formattedTime), "%c", timeInfo); printf("Formatted date and time: %s\n", formattedTime); return 0; }
输出
上述代码产生以下结果:
Current LC_TIME locale: en_US.UTF-8 Formatted date and time: Mon 20 May 2024 05:48:37 AM UTC
广告