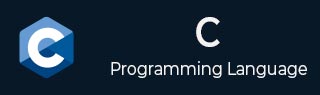
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - setvbuf() 函数
C 库的 setvbuf() 函数用于控制文件流的缓冲。此函数可以设置缓冲模式,并可选地为流指定缓冲区。
语法
以下是 C 库 setvbuf() 函数的语法:
int setvbuf(FILE *stream, char *buffer, int mode, size_t size);
参数
此函数接受以下参数:
- stream: 指向 FILE 对象的指针,用于标识打开的文件流。
- buffer: 指向将用作缓冲区的字符数组的指针。如果 buffer 为 NULL,则函数将自动分配缓冲区。
- mode: 指定缓冲模式的整数。它可以是以下之一:
- _IOFBF: 全缓冲。当缓冲区满时写入数据。
- _IOLBF: 行缓冲。当遇到换行符时写入数据。
- _IONBF: 无缓冲。立即写入数据。
- size: 缓冲区的大小(以字节为单位)。如果 buffer 为 NULL,则忽略此参数。
返回值
函数成功返回 0,失败返回非零值。
示例 1:使用自定义缓冲区的全缓冲
在这里,我们为全缓冲设置了一个大小为 1024 字节的自定义缓冲区。当缓冲区满或文件关闭时,数据将写入文件。
以下是 C 库 setvbuf() 函数的示例。
#include <stdio.h> int main() { FILE *file = fopen("example1.txt", "w"); if (!file) { perror("Failed to open file"); return 1; } char buffer[1024]; if (setvbuf(file, buffer, _IOFBF, sizeof(buffer)) != 0) { perror("Failed to set buffer"); return 1; } fputs("This is a test string.", file); fclose(file); return 0; }
输出
以上代码在文件关闭时将“This is a test string.”写入 example1.txt。
This is a test string.
示例 2:无缓冲
此示例禁用缓冲,这意味着数据将立即写入文件。
#include <stdio.h> int main() { FILE *file = fopen("example3.txt", "w"); if (!file) { perror("Failed to open file"); return 1; } if (setvbuf(file, NULL, _IONBF, 0) != 0) { perror("Failed to set buffer"); return 1; } fputs("This is a test string.", file); fputs("This is another string.", file); fclose(file); return 0; }
输出
执行以上代码后,它会立即将“This is a test string.This is another string.”写入 example3.txt,因为每次 fputs 调用都会进行写入。
This is a test string.This is another string.
广告