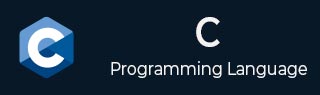
- C标准库
- C库 - 首页
- C库 - <assert.h>
- C库 - <complex.h>
- C库 - <ctype.h>
- C库 - <errno.h>
- C库 - <fenv.h>
- C库 - <float.h>
- C库 - <inttypes.h>
- C库 - <iso646.h>
- C库 - <limits.h>
- C库 - <locale.h>
- C库 - <math.h>
- C库 - <setjmp.h>
- C库 - <signal.h>
- C库 - <stdalign.h>
- C库 - <stdarg.h>
- C库 - <stdbool.h>
- C库 - <stddef.h>
- C库 - <stdio.h>
- C库 - <stdlib.h>
- C库 - <string.h>
- C库 - <tgmath.h>
- C库 - <time.h>
- C库 - <wctype.h>
C库 - sinh() 函数
C库函数`sinh()`,类型为double,接受参数x,返回双曲正弦值。
此函数在复数计算中非常有用,尤其是在处理复指数的虚部时。我们也可以在数值方法中使用此函数。
语法
以下是C库函数`sinh()`的语法:
double sinh(double x)
参数
此函数只接受一个参数。
x − 这是一个浮点值。
返回值
此函数返回x的双曲正弦值。
示例1
以下是一个基本的C库程序,用于演示`sinh()`函数。
#include <stdio.h> #include <math.h> int main () { double x, ret; x = 0.5; ret = sinh(x); printf("The hyperbolic sine of %lf is %lf degrees", x, ret); return(0); }
输出
以上代码产生以下输出:
The hyperbolic sine of 0.500000 is 0.521095 degrees
示例2
该程序对浮点值数组进行操作,通过循环计算双曲正弦值。
#include <stdio.h> #include <math.h> int main() { double val[] = {11.0, 21.0, 31.0, 41.0}; int num_val = sizeof(val) / sizeof(val[0]); printf("Hyperbolic Sine values:\n"); for (int i = 0; i < num_val; ++i) { double res = sinh(val[i]); printf("sinh(%.2f) = %.4f\n", val[i], res); } return 0; }
输出
执行以上代码后,我们得到以下结果:
Hyperbolic Sine values: sinh(11.00) = 29937.0708 sinh(21.00) = 659407867.2416 sinh(31.00) = 14524424832623.7129 sinh(41.00) = 319921746765027456.0000
示例3
在这个程序中,我们使用梯形法则进行数值积分来计算双曲正弦值。
#include <stdio.h> #include <math.h> // Function to calculate the hyperbolic sine double sinh(double x) { return (exp(x) - exp(-x)) / 2.0; } // Numerical integration using the trapezium rule double integrate_sinh(double a, double b, int num_intervals) { double h = (b - a) / num_intervals; double integral = 0.0; for (int i = 0; i < num_intervals; ++i) { double x0 = a + i * h; double x1 = a + (i + 1) * h; integral += (sinh(x0) + sinh(x1)) * h / 2.0; } return integral; } int main() { // Lower limit of integration double a = 0.0; // Upper limit of integration double b = 2.0; // Number of intervals for numerical integration int num_intervals = 1000; double result = integrate_sinh(a, b, num_intervals); printf("Integral of sinh(x) from %.2lf to %.2lf = %.6lf\n", a, b, result); return 0; }
输出
执行代码后,我们得到以下结果:
Integral of sinh(x) from 0.00 to 2.00 = 2.762197
广告