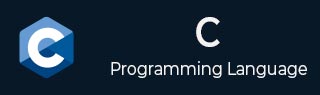
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - strcat() 函数
C 库 strcat() 函数接受两个指针变量作为参数(例如 dest、src),并将 src 指向的字符串追加到 dest 指向的字符串末尾。此函数仅连接字符串数据类型。我们不能使用任何其他数据类型,例如 int、float、char 等。
语法
以下是 C 库 strcat() 函数的语法 -
char *strcat(char *dest_str, const char *src_str)
参数
此函数接受以下参数 -
dest_str - 这是指向目标数组的指针,该数组应包含 C 字符串,并且应足够大以容纳连接后的结果字符串。
src_str - 要追加的字符串。这应该不与目标重叠。
返回值
此函数返回指向结果字符串(即 dest)的指针。
请注意,该函数在运行时动态地组合字符串。
示例 1
以下是说明使用 strcat() 函数进行字符串连接的代码片段的基本 C 库程序。
#include <stdio.h> #include <string.h> int main() { char dest_str[50] = "C "; const char src_str[] = "JAVA"; // Concatenate src_str to dest_str strcat(dest_str, src_str); // The result store in destination string printf("%s", dest_str); return 0; }
输出
执行上述代码后,我们将获得以下输出 -
Final destination string : |This is destinationThis is source|
示例 2
在以下示例中,我们使用 strcpy() 以及 strcat() 函数,将新字符串 (“point”) 与现有字符串 (“tutorials”) 合并。
#include <stdio.h> #include <string.h> int main() { char str[100]; // Copy the first string to the variable strcpy(str, "Tutorials"); // String concatenation strcat(str, "point"); // Show the result printf("%s\n", str); return 0; }
输出
上述代码产生以下输出 -
Tutorialspoint
示例 3
虽然连接两个不同的字符串始终需要两个参数,但这里我们使用整数作为 strcat() 的参数,这会导致错误。
#include <stdio.h> #include <string.h> int main() { int x = 20; int y = 24; strcat(x, y); printf("%d", x); return 0; }
输出
执行上述代码后,我们将获得以下结果 -
main.c: In function ‘main’: main.c:7:12: warning: passing argument 1 of ‘strcat’ makes pointer from integer without a cast [-Wint-conversion] 7 | strcat(x, y); | ^ | | | int In file included from main.c:2: /usr/include/string.h:149:39: note: expected ‘char * restrict’ but argument is of type ‘int’ 149 | extern char *strcat (char *__restrict __dest, const char *__restrict __src) | ~~~~~~~~~~~~~~~~~^~~~~~ main.c:7:15: warning: passing argument 2 of ‘strcat’ makes pointer from integer without a cast [-Wint-conversion] 7 | strcat(x, y); | ^ | | | int In file included from main.c:2: /usr/include/string.h:149:70: note: expected ‘const char * restrict’ but argument is of type ‘int’ 149 | extern char *strcat (char *__restrict __dest, const char *__restrict __src) |
广告