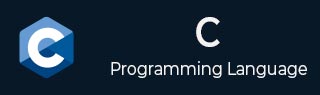
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - strcmp() 函数
C 库的 strcmp() 函数用于比较两个字符串。它逐个字符检查字符串,直到找到差异或到达其中一个字符串的末尾。此外,字符串比较基于 ASCII 值。
语法
以下是 C 库 strcmp() 函数的语法:
strcmp(const char *str_1, const char *str_2)
参数
此函数接受以下参数:
str_1 − 此参数定义要比较的第一个字符串。
str_2 − 这是与第一个/前一个字符串进行比较的第二个字符串。
返回值
此函数返回一个整数,其值为:
零,如果字符串相等。
负数,如果第一个字符串按字典顺序小于第二个字符串。
正数,如果第一个字符串按字典顺序大于第二个字符串。
示例 1
在此示例中,我们演示了使用 strcmp() 函数进行字符串字符比较。
#include <stdio.h> #include <string.h> int main() { char str1[] = "abcd", str2[] = "abcd"; int res; // Compare the strings str1 and str2 res = strcmp(str1, str2); printf("strcmp(str1, str2) = %d\n", res); return 0; }
输出
以上代码产生以下结果:
strcmp(str1, str3) = 0
示例 2
使用 strcmp() 和条件语句检查给定的字符串是否区分大小写。
#include <stdio.h> #include <string.h> int main() { char str1[] = "Tutorials"; char str2[] = "tutorials"; // Compare the strings int result = strcmp(str1, str2); if (result == 0) { printf("Strings are equal(case-sensitive)\n"); } else { printf("Strings are not equal(case-insensitive).\n"); } return 0; }
输出
执行以上代码后,我们得到以下结果:
Strings are not equal(case-insensitive).
示例 3
以下示例创建不同大小的字符串,并使用 strcmp() 检查其比较结果。
#include <stdio.h> #include <string.h> int main() { char str_1[] = "BOOK"; char str_2[] = "UNIVERSITY"; int res = strcmp(str_1, str_2); if (res == 0) { printf("The strings are equal.\n"); } else if (res < 0) { printf("Str_1 is less than str_2.\n"); } else { printf("Str_1 is greater than str_2.\n"); } return 0; }
输出
执行以上代码后,我们得到以下结果:
Str_1 is less than str_2.
示例 4
在这里,我们使用 strcmp() 来比较两个不同的字符串,并借助字典序。
#include <stdio.h> #include <string.h> int main { char str1[] = "text"; char str2[] = "notebook"; int result = strcmp(str1, str2); if (result < 0) { printf("'%s' comes before '%s' lexicographically.\n", str1, str2); } else if (result > 0) { printf("'%s' comes after '%s' lexicographically.\n", str1, str2); } else { printf("Strings are equal.\n"); } return 0; }
解释
在此代码中,str1 ("text") 按字典顺序在 str2 ("Notebook") 之前。strcmp() 函数返回一个负值。
以上代码产生以下结果:
'text' comes after 'notebook' lexicographically.
广告