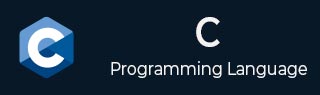
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - strcpy() 函数
C 库的 strcpy() 函数接受两个参数,将 src 指向的字符串复制到 dest。此函数对于维护和升级旧系统至关重要。
语法
以下是 C 库 strcpy() 函数的语法:
char *strcpy(char *dest, const char *src)
参数
此函数接受以下参数:
dest - 这是指向目标数组的指针,内容将被复制到该数组。
src - 这是要复制的字符串。
确保目标数组有足够的空间容纳源字符串,包括空终止符。
返回值
此函数返回指向目标字符串 dest 的指针。
示例 1
以下是一个基本的 C 程序,演示了 strcpy() 函数的使用。
#include <stdio.h> #include <string.h> int main () { char src[40]; char dest[100]; memset(dest, '\0', sizeof(dest)); strcpy(src, "This is tutorialspoint.com"); strcpy(dest, src); printf("Final copied string : %s\n", dest); return(0); }
输出
执行上述代码后,我们将获得以下输出:
Final copied string : This is tutorialspoint.com
示例 2
下面的示例展示了自定义字符串复制,不使用 strcpy()。
#include <stdio.h> void customStrcpy(char* dest, const char* src) { while (*src) { *dest = *src; dest++; src++; } *dest = '\0'; } int main() { char source[] = "string Copy!"; char destination[20]; customStrcpy(destination, source); printf("The custom copied string: %s\n", destination); return 0; }
输出
执行上述代码后,我们将获得以下输出:
The custom copied string: string Copy!
示例 3
在这里,我们定义了两个字符串变量 - source 和 destination。然后应用 strcpy(),它接受这两个变量来确定字符串复制的结果。
#include <stdio.h> #include <string.h> int main() { char source[] = "Hello, World!"; char destination[20]; strcpy(destination, source); printf("The result of copied string: %s\n", destination); return 0; }
输出
上述代码产生以下输出:
The result of copied string: Hello, World!
广告