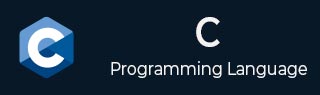
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - strftime() 函数
C 库的 strftime() 函数用于将日期和时间格式化为字符串。它允许用户设置自定义的日期和时间表示形式,这使得该函数更有价值。
在这里,size_t strftime(char *str, size_t maxsize, const char *format, const struct tm *timeptr) 根据 format 中定义的格式化规则格式化结构 timeptr 中表示的时间,并将其存储到 str 中。
语法
以下是 C 库 strftime() 函数的语法:
size_t strftime(char *str, size_t maxsize, const char *format, const struct tm *timeptr)
参数
此函数接受以下参数:
- str - 这是指向目标数组的指针,结果 C 字符串将复制到该数组中。
- maxsize - 这是要复制到 str 的最大字符数。
- format - 这是包含任意组合的常规字符和特殊格式说明符的 C 字符串。这些格式说明符将由函数替换为相应的数值,以表示 tm 中指定的时间。格式说明符如下:
说明符 | 替换为 | 示例 |
---|---|---|
%a | 缩写星期几名称 | Sun |
%A | 完整星期几名称 | Sunday |
%b | 缩写月份名称 | Mar |
%B | 完整月份名称 | March |
%c | 日期和时间表示形式 | Sun Aug 19 02:56:02 2012 |
%d | 月份中的日期 (01-31) | 19 |
%H | 24 小时制的小时 (00-23) | 14 |
%I | 12 小时制的小时 (01-12) | 05 |
%j | 一年中的日期 (001-366) | 231 |
%m | 月份的十进制数 (01-12) | 08 |
%M | 分钟 (00-59) | 55 |
%p | AM 或 PM 表示 | PM |
%S | 秒 (00-61) | 02 |
%U | 以第一个星期日作为第一周的第一天计算的星期数 (00-53) | 33 |
%w | 以星期日为 0 的星期几的十进制数 (0-6) | 4 |
%W | 以第一个星期一作为第一周的第一天计算的星期数 (00-53) | 34 |
%x | 日期表示形式 | 08/19/12 |
%X | 时间表示形式 | 02:50:06 |
%y | 年份的后两位数字 (00-99) | 01 |
%Y | 年份 | 2012 |
%Z | 时区名称或缩写 | CDT |
%% | 一个 % 符号 | % |
timeptr - 这是指向 tm 结构的指针,该结构包含分解成其组成部分的日历时间,如下所示:
struct tm { int tm_sec; /* seconds, range 0 to 59 */ int tm_min; /* minutes, range 0 to 59 */ int tm_hour; /* hours, range 0 to 23 */ int tm_mday; /* day of the month, range 1 to 31 */ int tm_mon; /* month, range 0 to 11 */ int tm_year; /* The number of years since 1900 */ int tm_wday; /* day of the week, range 0 to 6 */ int tm_yday; /* day in the year, range 0 to 365 */ int tm_isdst; /* daylight saving time */ };
返回值
如果结果 C 字符串的字符数少于 size 个字符(包括终止空字符),则返回复制到 str 中的字符总数(不包括终止空字符)。否则,它返回零。
示例 1
以下是基本 C 程序库,用于演示 strftime() 函数,该函数将生成日期和时间。
#include <stdio.h> #include <time.h> int main () { time_t rawtime; struct tm *info; char buffer[80]; time( &rawtime ); info = localtime( &rawtime ); strftime(buffer,80,"%x - %I:%M%p", info); printf("Formatted date and time : |%s|\n", buffer ); return(0); }
输出
执行上述代码后,我们将得到以下结果:
Formatted date and time : |05/15/24 - 08:22AM|
示例 2
在使用 locatime() 函数时,它会将当前时间转换为本地时间,并将结果以字符串格式返回。
#include <stdio.h> #include <time.h> #define Size 50 int main() { time_t t; struct tm *tmp; char MY_TIME[Size]; // Get the current time time(&t); // Convert to local time tmp = localtime(&t); // Format the date and time strftime(MY_TIME, sizeof(MY_TIME), "%x - %I:%M%p", tmp); printf("Formatted date & time: %s\n", MY_TIME); return 0; }
输出
以上代码生成以下结果:
Formatted date & time: 05/15/24 - 08:26AM
示例 3
C 程序使用 localtime() 和 strftime() 函数打印当前日期和时间。
#include<stdio.h> #include<time.h> int main() { time_t anytime; struct tm *current; char time_str[64]; time(&anytime); current = localtime(&anytime); strftime(time_str, 64, "%A, %B %d, %Y", current); printf("Today is %s\n", time_str); return 0; }
输出
执行代码后,我们将得到以下结果:
Today is Wednesday, May 15, 2024
广告