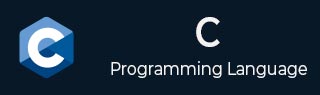
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - strncpy() 函数
C 库函数 `strncpy()` 接受三个参数 (dest, src, n),它将 `src` 指向的字符串中最多 `n` 个字符复制到 `dest`。如果 `src` 的长度小于 `n`,则 `dest` 的剩余部分将用空字节填充。
语法
以下是 C 库函数 `strncpy()` 的语法:
char *strncpy(char *dest, const char *src, size_t n)
参数
此函数接受以下参数:
dest − 这是指向目标数组的指针,内容将被复制到该数组。
src − 这是要复制的字符串。
n − 要从源复制的字符数。
返回值
此函数返回指向已复制字符串的指针。
示例 1
以下是一个基本的 C 库程序,演示了 `strncpy()` 函数的用法。
#include <stdio.h> #include <string.h> int main () { char src[40]; char dest[12]; memset(dest, '\0', sizeof(dest)); strcpy(src, "This is tutorialspoint.com"); strncpy(dest, src, 10); printf("Final copied string : %s\n", dest); return(0); }
输出
执行上述代码后,我们将得到以下结果:
Final copied string : This is tu
示例 2
下面的示例演示了使用自定义字符串复制和固定长度。
#include <stdio.h> #include <string.h> void customStrncpy(char* dest, const char* src, size_t n) { size_t i; for (i = 0; i < n && src[i] != '\0'; i++) { dest[i] = src[i]; } while (i < n) { // Fill remaining characters with null terminators dest[i] = '\0'; i++; } } int main() { char source[] = "String Copy!"; // Fixed length char destination[15]; customStrncpy(destination, source, 10); printf("Custom copied string: %s\n", destination); return 0; }
输出
执行上述代码后,我们将得到以下结果:
Custom copied string: String Cop
示例 3
在这个程序中,`strncpy()` 将源字符串中的固定长度子字符串复制到目标字符串中。
#include <stdio.h> #include <string.h> int main() { char source[] = "Hello, Note!"; // Fixed length char destination[10]; // Copy "Note!" strncpy(destination, source + 7, 4); printf("Copied substring: %s\n", destination); return 0; }
输出
执行上述代码后,我们将得到以下结果:
Copied substring: Note
广告