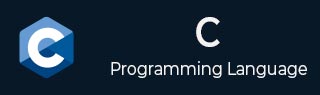
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - strrchr() 函数
C 库的 strrchr() 函数接受两个参数 - 在参数 str 指向的字符串中搜索字符 c(一个无符号字符)的最后一次出现。
以下是一些突显其重要性的关键点:
- 此函数用于查找指定字符的最后一次出现。
- 主要操作是文件路径操作、解析 URL 和处理数据格式。
- 它节省了时间并减少了错误。
语法
以下是 C 库函数 strrchr() 的语法:
char *strrchr(const char *str, int c)
参数
此函数接受两个参数:
str - 这是 C 字符串。
c - 这是要查找的字符。它作为其 int 提升传递,但在内部转换回 char。
返回值
此函数返回指向 str 中字符最后一次出现的指针。如果未找到该值,则该函数返回空指针。
示例 1
以下是一个基本的 C 库程序,它展示了 strrchr() 函数的使用。
#include <stdio.h> #include <string.h> int main () { int len; const char str[] = "https://tutorialspoint.com"; const char ch = '.'; char *ret; ret = strrchr(str, ch); printf("String after |%c| is - |%s|\n", ch, ret); return(0); }
输出
以上代码产生以下结果:
String after |.| is - |.com|
示例 2
下面的程序使用 strrchr() 在给定字符串的子字符串中搜索字符。
#include <stdio.h> #include <string.h> int main() { const char str[] = "Hello, World!"; const char ch = 'W'; // Search in the substring "World!" char* ptr = strrchr(str + 7, ch); if (ptr) { printf("'%c' found at position %ld\n", ch, ptr - str); } else { printf("'%c' not found in the substring\n", ch); } return 0; }
输出
执行以上代码后,我们得到以下结果:
'W' found at position 7
示例 3
在本例中,我们将使用 strrchr() 查找字符的最后一次出现。
#include <stdio.h> #include <string.h> int main() { const char str[] = "Tutorialspoint"; const char ch = 'n'; char* ptr = strrchr(str, ch); if (ptr) { printf("Last occurrence of '%c' in \"%s\" is at index %ld\n", ch, str, ptr - str); } else { printf("'%c' is not present in \"%s\"\n", ch, str); } return 0; }
输出
执行代码后,我们得到以下结果:
Last occurrence of 'n' in "Tutorialspoint" is at index 12
广告