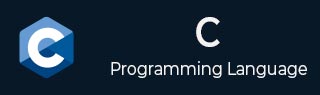
- C标准库
- C库 - 首页
- C库 - <assert.h>
- C库 - <complex.h>
- C库 - <ctype.h>
- C库 - <errno.h>
- C库 - <fenv.h>
- C库 - <float.h>
- C库 - <inttypes.h>
- C库 - <iso646.h>
- C库 - <limits.h>
- C库 - <locale.h>
- C库 - <math.h>
- C库 - <setjmp.h>
- C库 - <signal.h>
- C库 - <stdalign.h>
- C库 - <stdarg.h>
- C库 - <stdbool.h>
- C库 - <stddef.h>
- C库 - <stdio.h>
- C库 - <stdlib.h>
- C库 - <string.h>
- C库 - <tgmath.h>
- C库 - <time.h>
- C库 - <wctype.h>
C库 - strtol() 函数
C 的stdlib库 strtol() 函数用于根据给定的基数将字符串转换为长整型数字。基数必须在 2 到 32(包括 2 和 32)之间,或者为特殊值 0。
语法
以下是C库中strtol()函数的语法:
long int strtol(const char *str, char **endptr, int base)
参数
此函数接受以下参数:
-
str − 要转换为长整型值的字符串。
-
endptr − 指向字符指针的指针,用于存储数值后第一个字符的指针。
-
base − 表示数字系统的基数(例如,10 表示十进制,16 表示十六进制)。
返回值
此函数返回转换后的长整型值。如果输入字符串无效,则返回 0。
示例 1
在这个例子中,我们创建了一个基本的C程序来演示如何使用strtol()函数。
#include <stdio.h> #include <stdlib.h> int main() { const char *str = "12345abc"; char *endptr; long int num; // Convert the string to a long integer num = strtol(str, &endptr, 10); if (endptr == str) { printf("No digits were found.\n"); } else if (*endptr != '\0') { printf("Invalid character: %c\n", *endptr); } else { printf("The number is: %ld\n", num); } return 0; }
输出
以下是输出:
Invalid character: a
示例 2
下面的示例使用 strtol() 函数将十进制数字字符串转换为长十进制整数。
#include <stdio.h> #include <stdlib.h> int main() { const char *str = "12205"; char *endptr; long int num; // Convert the string to a long integer num = strtol(str, &endptr, 10); //display the long integer number printf("The number is: %ld\n", num); return 0; }
输出
以下是输出:
The number is: 12205
示例 3
让我们再创建一个示例,使用 strtol() 函数将数字转换为长十六进制数。
#include <stdio.h> #include <stdlib.h> int main() { const char *str = "2024 tutorialspoint"; char *endptr; long int num; // Convert the string to a long integer num = strtol(str, &endptr, 16); //display the long hexadecimal integer number printf("The hexadecimal number is: %ld\n", num); return 0; }
输出
以下是输出:
The hexadecimal number is: 8228
广告