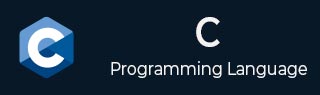
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - strtoul() 函数
C 的stdlib 库 strtoul() 函数根据给定的基数将字符串的初始部分转换为无符号长整型数字,该基数必须在 2 到 32(包括 2 和 32)之间,或者为特殊值 0。
当整数的大小超出普通整数类型的范围时,使用 strtoul() 函数。它也用于转换表示不同基数的数字的字符串,例如将二进制转换为十六进制。
语法
以下是 strtoul() 函数的 C 库语法:
unsigned long int strtoul(const char *str, char **endptr, int base)
参数
此函数接受以下参数:
-
str - 要转换为无符号长整型值的字符串。
-
endptr - char* 类型的对象的引用,函数将其值设置为 str 中数值后的下一个字符。
-
base - 表示数字系统的基数(例如,10 表示十进制,16 表示十六进制,或 0 表示特殊情况)。
返回值
此函数返回转换后的无符号长整型值。如果输入字符串无效,则返回 0。
示例 1
在此示例中,我们创建一个基本的 C 程序来演示如何使用 strtoul() 函数。
#include <stdio.h> #include <stdlib.h> int main() { const char *str = "12532abc"; char *endptr; unsigned long int value; //use the strtoul() function value = strtoul(str, &endptr, 0); printf("The unsigned long integer is: %lu\n", value); printf("String part is: %s", endptr); return 0; }
输出
以下是输出:
The unsigned long integer is: 12532 String part is: abc
示例 2
以下示例使用 strtoul() 函数将字符串的数字部分转换为无符号长十六进制整数。
#include <stdio.h> #include <stdlib.h> int main() { // initializing the string char str[150] = "202405Tutorialspoint India"; // reference pointer char* endptr; unsigned long int value; // finding the unsigned long // integer with base 16 value = strtoul(str, &endptr, 16); // displaying the unsigned number printf("The unsigned long integer is: %lu\n", value); printf("String in str is: %s", endptr); return 0; }
输出
以下是输出:
The unsigned long integer is: 2106373 String in str is: Tutorialspoint India
示例 3
让我们再创建一个示例,我们尝试将不包含任何数字字符的字符串转换为无符号长十进制数字。
#include <stdio.h> #include <stdlib.h> int main() { const char *str = "abcde"; char *endptr; unsigned long int value; //use the strtoul() funcion value = strtoul(str, &endptr, 10); if (value == 0 && endptr == str) { printf("No numeric characters were found in the string.\n"); } printf("unsigned long integer is: %lu\n", value); printf("String part is: %s", endptr); return 0; }
输出
以下是输出:
No numeric characters were found in the string. unsigned long integer is: 0 String part is: abcde
广告