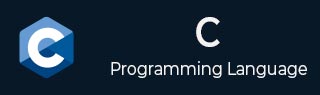
- C标准库
- C库 - 首页
- C库 - <assert.h>
- C库 - <complex.h>
- C库 - <ctype.h>
- C库 - <errno.h>
- C库 - <fenv.h>
- C库 - <float.h>
- C库 - <inttypes.h>
- C库 - <iso646.h>
- C库 - <limits.h>
- C库 - <locale.h>
- C库 - <math.h>
- C库 - <setjmp.h>
- C库 - <signal.h>
- C库 - <stdalign.h>
- C库 - <stdarg.h>
- C库 - <stdbool.h>
- C库 - <stddef.h>
- C库 - <stdio.h>
- C库 - <stdlib.h>
- C库 - <string.h>
- C库 - <tgmath.h>
- C库 - <time.h>
- C库 - <wctype.h>
C库 - tanh() 函数
C库函数`tanh()`接受类型为double的参数(x),并返回x的双曲正切值。
传递给tanh()的参数可以是任何实数,无论是正数还是负数。tanh()函数在现实生活中的应用包括:神经网络、信号处理、统计和数据分析。
语法
以下是C库函数`tanh()`的语法:
double tanh(double x)
参数
此函数只接受一个参数:
x - 这是一个浮点值。
返回值
此函数返回x的双曲正切值。
示例1
以下是一个C库程序,演示`tanh()`函数的使用。
#include <stdio.h> #include <math.h> int main () { double x, ret; x = 0.5; ret = tanh(x); printf("The hyperbolic tangent of %lf is %lf degrees", x, ret); return(0); }
输出
以上代码产生以下结果:
The hyperbolic tangent of 0.500000 is 0.462117 degrees
示例2
在这个程序中,角度设置为30度作为输入。因此,我们可以调整`angle_degrees`的值来探索不同的角度,并观察它们对应的双曲正切值。
#include <stdio.h> #include <math.h> int main() { // angle in degree double angle_deg = 30.0; // Convert degrees to radians double angle_radians = angle_deg * (M_PI / 180.0); // Calculate tanh double res = tanh(angle_radians); printf("tanh(%.2lf degrees) = %.4lf\n", angle_deg, res); return 0; }
输出
执行以上代码后,我们得到以下结果:
tanh(30.00 degrees) = 0.4805
示例3
下面的例子描述了使用tanh()逼近e^x的方法。这里,我们定义了一个自定义函数`my_exp()`,它使用公式`ex = (1 + tanh(x/2)) / (1 - tanh(x/2))`来返回结果。
#include <stdio.h> #include <math.h> double my_exp(double x) { return (1.0 + tanh(x / 2.0)) * (1.0 - tanh(x / 2.0)); } int main() { double x = 2.0; double apx_exp = my_exp(x); printf("Approximate e^%.2lf = %.6lf\n", x, apx_exp); return 0; }
输出
执行以上代码后,我们得到以下结果:
Approximate e^2.00 = 0.419974 Approximate e^2.00 = 0.419974
广告