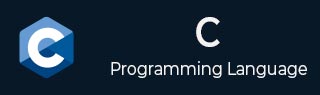
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - towlower() 函数
C 的wctype 库 towlower() 函数用于将给定的宽字符转换为小写字符(如果可能)。
此函数可用于不区分大小写的比较、文本规范化或用户输入处理。
语法
以下是 towlower() 函数的 C 库语法:
wint_t towlower( wint_t wc );
参数
此函数接受单个参数:
-
wc − 一个类型为 'wint_t' 的宽字符,将被转换为小写。
返回值
如果宽字符已更改为小写字符,则此函数返回小写字符;否则返回未更改的字符(如果它已经是小写字符或不是字母字符)。
示例 1
以下是一个基本的 C 示例,演示了 towlower() 函数的使用。
#include <wchar.h> #include <wctype.h> #include <stdio.h> int main() { // wide character wchar_t wc = L'G'; // convert to lowercase wint_t lower_wc = towlower(wc); wprintf(L"The lowercase equivalent of %lc is %lc\n", wc, lower_wc); return 0; }
输出
以下是输出:
The lowercase equivalent of G is g
示例 2
我们创建一个 C 程序来比较字符串是否相等。 使用 towlower() 转换为小写后进行比较。如果两个字符串相等,则表示不区分大小写,否则区分大小写。
#include <wchar.h> #include <wctype.h> #include <stdio.h> #include <string.h> int main() { wchar_t str1[] = L"Hello World"; wchar_t str2[] = L"hello world"; // flag value int equal = 1; // comepare both string after compare for (size_t i = 0; i < wcslen(str1); ++i) { if (towlower(str1[i]) != towlower(str2[i])) { // strings are not equal equal = 0; break; } } if (equal) { wprintf(L"The strings are equal (case insensitive).\n"); } else { wprintf(L"The strings are not equal.\n"); } return 0; }
输出
以下是输出:
The strings are equal (case insensitive).
示例 3
下面的示例将宽字符规范化为小写字符。
#include <wchar.h> #include <wctype.h> #include <stdio.h> int main() { // Wide string wchar_t text[] = L"Normalize THIS Tutorialspoint!"; size_t length = wcslen(text); wprintf(L"Original text: %ls\n", text); // Normalize the text to lowercase for (size_t i = 0; i < length; i++) { text[i] = towlower(text[i]); } wprintf(L"Normalized text: %ls\n", text); return 0; }
输出
以下是输出:
Original text: Normalize THIS Tutorialspoint! Normalized text: normalize this tutorialspoint!
c_library_wctype_h.htm
广告