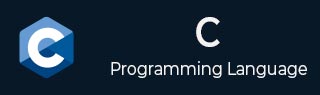
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - wcsftime() 函数
C 库的 wcsftime() 函数根据指定的格式将日期和时间信息转换为宽字符字符串。在打印日期时,它使用 wprintf() 函数。此函数用于使用宽字符进行格式化输出。它允许用户将一系列宽字符和值打印到标准输出。
语法
以下是 C 库 wcsftime() 函数的语法 -
wcsftime(buffer, int_value, L"The current Date: %A, %F %I:%M %p", timeinfo); and wprintf(L"%ls\n", buffer);
参数
此函数接受以下参数 -
1. buffer:指向输出将存储到的宽字符数组的第一个元素的指针。
2. int_valuie:要写入的最大宽字符数(缓冲区的大小)。
3. format:指向一个以 null 结尾的宽字符字符串的指针,该字符串指定转换的格式,以下是日期和时间格式化中使用的常用转换说明符列表 -
- %A:完整的工作日名称(例如,“星期日/星期一/星期二等”)。
- %F:完整日期,格式为“YYYY-MM-DD”(例如,“2024-05-22”)。
- %H:小时(24 小时制),以十进制数字表示(例如,“19”)。
- %M:分钟,以十进制数字表示(例如,“30”)。
- %I:%M %p:小时(12 小时制)和分钟,后跟 AM/PM 指示符。
- %S:秒,以十进制数字表示(例如,“45”)。
4. timeinfo:指向包含本地时间信息的 struct tm 的指针。
返回类型
程序成功时,函数返回复制到字符串中的宽字符总数,不包括终止 null 宽字符。如果输出超过最大大小,则函数返回零。
示例 1
以下是使用 wcsftime() 函数显示当前日期和时间并使用自定义格式的基本 C 库程序。
#include <stdio.h> #include <wchar.h> #include <time.h> int main() { time_t demotime; struct tm* timeinfo; wchar_t buffer[80]; time(&demotime); timeinfo = localtime(&demotime); // Custom formatting of time wcsftime(buffer, 80, L"The current Date: %A, %F %I:%M %p", timeinfo); wprintf(L"%ls\n", buffer); return 0; }
输出
执行上述代码后,我们将获得以下输出 -
The current Date: Wednesday, 2024-05-22 01:38 PM
示例 2
以下示例使用各种函数以 24 小时制格式化本地时间。
#include <stdio.h> #include <wchar.h> #include <locale.h> #include <time.h> int main() { time_t rawtime; struct tm* timeinfo; wchar_t buffer[80]; time(&rawtime); timeinfo = localtime(&rawtime); // Set the locale category setlocale(LC_ALL, "ja_JP.utf8"); // Custom Formatting to 24-hour clock wcsftime(buffer, 80, L"現在の日時: %A、%F %H:%M", timeinfo); // Display the result wprintf(L"%ls\n", buffer); return 0; }
输出
执行代码后,我们将获得以下输出 -
?????: Wednesday?2024-05-22 13:42
示例 3
要获取今天的日期,wcsftime() 格式设置转换说明符的自定义设置并显示结果。
#include <stdio.h> #include <time.h> #include <wchar.h> int main(void) { struct tm* timeinfo; wchar_t dest[100]; time_t temp; size_t r; temp = time(NULL); timeinfo = localtime(&temp); r = wcsftime(dest, sizeof(dest), L" Today is %A, %b %d.\n Time: %I:%M %p", timeinfo); printf("%zu is the placed characters in the string:\n\n%ls\n", r, dest); return 0; }
输出
以上代码产生以下输出 -
44 The placed characters in the string: Today is Wednesday, May 22. Time: 02:28 PM
广告