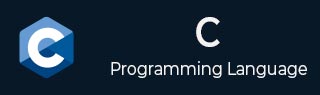
- C 标准库
- C 库 - 首页
- C 库 - <assert.h>
- C 库 - <complex.h>
- C 库 - <ctype.h>
- C 库 - <errno.h>
- C 库 - <fenv.h>
- C 库 - <float.h>
- C 库 - <inttypes.h>
- C 库 - <iso646.h>
- C 库 - <limits.h>
- C 库 - <locale.h>
- C 库 - <math.h>
- C 库 - <setjmp.h>
- C 库 - <signal.h>
- C 库 - <stdalign.h>
- C 库 - <stdarg.h>
- C 库 - <stdbool.h>
- C 库 - <stddef.h>
- C 库 - <stdio.h>
- C 库 - <stdlib.h>
- C 库 - <string.h>
- C 库 - <tgmath.h>
- C 库 - <time.h>
- C 库 - <wctype.h>
- C 标准库资源
- C 库 - 快速指南
- C 库 - 有用资源
- C 库 - 讨论
C 库 - va_start() 宏
C 的stdarg 库va_start() 宏允许访问命名参数parmN之后的可变参数。它用于初始化一个'va_list'变量,然后使用该变量检索传递给函数的其他参数。
在任何对 va_arg(可变参数)的调用之前,都应使用有效的 va_list(变量列表)对象ap的实例调用va_start。
此宏对于创建可变参数函数很有用,它允许我们创建一个可以接受可变数量参数的函数。它至少包含一个固定参数,后跟省略号(...)。
语法
以下是va_start()宏的 C 库语法:
void va_start(va_list ap, parmN)
参数
此宏接受以下参数:
-
ap - 它是一个 va_list 类型的变量,将由'va_start'初始化。此变量用于遍历参数列表。
parmN - 它是函数定义中最后一个命名参数的名称。
返回值
此宏不返回值。
示例 1
以下是演示va_start()用法的基本 C 示例。
#include <stdio.h> #include <stdarg.h> int sum(int count, ...) { va_list args; int tot = 0; // Set the va_list variable with the last fixed argument va_start(args, count); // Retrieve the arguments and calculate the sum for (int i = 0; i < count; i++) { tot = tot + va_arg(args, int); } // use the va_end to clean va_list variable va_end(args); return tot; } int main() { // Call the sum, with number of arguments printf("Sum of 3, 5, 7, 9: %d\n", sum(4, 3, 5, 7, 9)); printf("Sum of 1, 2, 3, 4, 5: %d\n", sum(5, 1, 2, 3, 4, 5)); return 0; }
输出
以下是输出:
Sum of 3, 5, 7, 9: 24 Sum of 1, 2, 3, 4, 5: 15
示例 2
在此示例中,我们使用va_start()来计算用户定义函数中传递的参数数量。
#include <stdio.h> #include <stdarg.h> int cnt(int count, ...) { va_list args; int num_of_arg = 0; // Set the va_list variable with the last fixed argument va_start(args, count); // Retrieve the arguments and count the arguments for (int i = 0; i < count; i++) { num_of_arg= num_of_arg + 1; } // use the va_end to clean va_list variable va_end(args); return num_of_arg; } int main() { // Call the sum, with number of arguments printf("Number of arguments: %d\n", cnt(4, 3, 5, 7, 9)); printf("Number of arguments: %d\n", cnt(5, 1, 2, 3, 4, 5)); return 0; }
输出
以下是输出:
Number of arguments: 4 Number of arguments: 5
示例 3
让我们看另一个例子,在这里我们创建一个函数,将可变数量的字符串连接到单个结果字符串中。
#include <stdio.h> #include <stdarg.h> #include <stdlib.h> #include <string.h> char* concatenate(int count, ...) { va_list args; int length = 0; // calculate length va_start(args, count); for (int i = 0; i < count; i++) { length = length + strlen(va_arg(args, char*)); } va_end(args); // Allocate memory for the result string char *res = (char*)malloc(length + 1); if (!res) { return NULL; } // Concatenate the strings // Initialize result as an empty string res[0] = '\0'; va_start(args, count); for (int i = 0; i < count; i++) { strcat(res, va_arg(args, char*)); } va_end(args); return res; } int main() { char *res = concatenate(3, "Hello, ", "tutorialspoint", "India"); if (res) { printf("%s\n", res); //free the alocated memory free(res); } return 0; }
输出
以下是输出:
Hello, tutorialspointIndia
广告