检查字符串是否可以使用qwerty键盘的同一行打印
简介
在本教程中,我们将检查输入字符串是否可以使用Qwerty键盘的同一行中的字符形成。任务是检查给定的字符串是否存在于Qwerty键盘的单行中。为了确定字符串是否可以使用Qwerty键盘的同一行打印,所有字符都应该在同一行中找到。我们使用set和unordered_set实现了一种方法来解决此任务。不同行的字符存储在不同的集合或无序集合中。将字符串字符与每个存储的行值进行比较。
Qwerty键盘是所有计算机、笔记本电脑和打印机的标准键盘。Qwerty键盘上的字符专门设计用于快速打字。
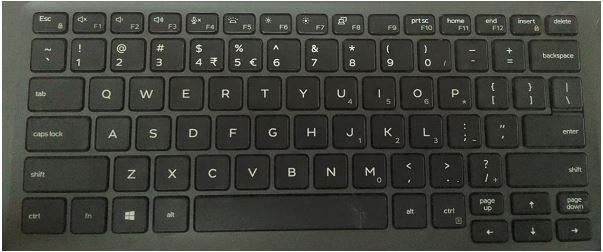
示例 1
String = "qwerty"
输出
Yes, the string exists in the same row of the qwerty keypad.
解释
输入字符串为“qwerty”,此字符串可以通过qwerty键盘第一行的字符形成。输入字符串的每个字符都存在于同一行中。因此,可以使用qwerty键盘的同一行打印该字符串。
示例 2
String = "hello"
输出
NO, the string does not exist in the same row of the qwerty keypad.
解释
输入字符串为“hello”,它无法使用qwerty键盘的同一行打印。输入字符串的字符存在于不同的行中。因此,输出为否。
C++库函数
Unordered_set - 它是C++中的一种数据结构。它是一种集合(有序和无序集合),它以无序的方式存储元素(不是以任何排序方式)。
unordered_set <data_type> unordered_set_name;
set - 它是用于存储特定数据类型的不同元素的容器。集合以某种排序顺序存储元素,并且由于其标识符,每个元素都是唯一的。它不允许重复元素。
set<data_type> set_name;
length() - 它是字符串类库函数,在<string>头文件中定义。它以字节为单位返回输入字符串的长度。
string_name.length();
set.count() - 它是集合的预定义函数。它计算值与集合存储的元素匹配的次数。当在集合中找到元素时,它返回1,否则返回0。
set_name.count();
算法
初始化输入字符串的值。
将每行的字符存储在不同的集合中。
使用if-else条件将字符串与集合的每个元素进行比较。
如果所有字符串字符都存在于同一集合中,则输出为是。
否则输出为否。
打印输出。
示例 1
我们使用多个集合在C++中实现了本教程的问题陈述。同一行的字符存储在一个集合中,并将元素存储在三个不同的集合中。将输入字符串的字符与每个集合中的元素进行比较。
#include <bits/stdc++.h> using namespace std; // user-defined function to check string exist in same row of the qwerty keypad int checkString(char c){ // storing element of the different row of the qwerty keypad in different sets set<char> row_1 = { '1', '2', '3', '4', '5', '6', '7', '8', '9', '0', '-', '=' }; set<char> row_2 = { 'Q', 'W', 'E', 'R', 'T', 'Y', 'U', 'I', 'O', 'P', '[', ']', 'q', 'w', 'e', 'r', 't', 'y', 'u', 'i', 'o', 'p' }; set<char> row_3 = { 'A', 'S', 'D', 'F', 'G','H', 'J', 'K', 'L', ';', ':', 'a', 's', 'd', 'f', 'g', 'h', 'j', 'k', 'l' }; set<char> row_4 = { 'Z', 'X', 'C', 'V', 'B', 'N', 'M', ',', '.', '/', 'z', 'x', 'c', 'v', 'b', 'n', 'm' }; if (row_1.count(c) > 0) return 1; else if (row_2.count(c) > 0) return 2; else if (row_3.count(c) > 0) return 3; else if (row_4.count(c) > 0) return 4; return 0; } // Function to check the input string can match the same row characters of the qwerty keypad bool checkStringExist(string s) { char c = s[0]; int rowValue = checkString(c); for (int x = 0; x < s.length(); x++) { c = s[x]; if (rowValue != checkString(c)) return false;} return true; } int main() { string s = "qwerty"; if (checkStringExist(s)) cout << "Yes, the string can be printed using the same row of the qwerty keypad."; else cout << "No, the string can be printed using the same row of the qwerty keypad."; return (0); }
输出
Yes, the string can be printed using the same row of the qwerty keypad.
示例 2
在本教程中C++实现的问题陈述中,我们使用多个unordered_set来存储Qwerty键盘不同行的值。所有行的字符都小写。如果字符串包含大写字符,则将其转换为小写。
#include <iostream> #include <unordered_set> #include <cctype> using namespace std; //user-defined function to check if string can be printed using the same roe of the qwerty keypad bool checkString(const string& s) { // different unordered_set to store elements unordered_set<char> row1 = {'q', 'w', 'e', 'r', 't', 'y', 'u', 'i', 'o', 'p'}; unordered_set<char> row2 = {'a', 's', 'd', 'f', 'g', 'h', 'j', 'k', 'l'}; unordered_set<char> row3 = {'z', 'x', 'c', 'v', 'b', 'n', 'm'}; // identifying the row of the string characters char stringChar = tolower(s[0]); unordered_set<char>* rowValues; if (row1.count(stringChar)) rowValues = &row1; else if (row2.count(stringChar)) rowValues = &row2; else if (row3.count(stringChar)) rowValues = &row3; else return false; // checking if all characters of the string exist in same row for (char c : s) { if (tolower(c) != stringChar && !rowValues->count(tolower(c))) return false; } return true; } //code controller int main() { string s = "Qwert"; // Predefined string //calling function if (checkString(s)) cout << "Yes, the string can be printed using the same row of the QWERTY keypad." << endl; else cout << "No, the string cannot be printed using the same row of the QWERTY keypad." << endl; return 0; }
输出
Yes, the string can be printed using the same row of the QWERTY keypad.
结论
我们已经到达本教程的结尾。我们已经实现了一种方法来检查输入字符串是否可以使用Qwerty键盘的同一行中的字符打印。只有当所有字符都存在于一行中时,才能从Qwerty键盘的同一行打印字符串。将不同行的值存储在集合和无序集合中,并比较字符串字符。我们使用演示来详细说明本教程的问题陈述的含义。