如何在Android的AsyncTask中返回布尔值?
介绍
AsyncTask是Android中用于执行一些后台长时间运行任务的类,例如从互联网后台加载图像。如果图像尺寸很大,则可以使用Android中的AsyncTask将图像作为后台任务从互联网加载。本文将介绍如何在Android的AsyncTask中返回布尔值。
实现
我们将创建一个简单的应用程序,其中我们将显示一个用于应用程序标题的TextView。然后,我们创建另一个TextView,用于显示任务正在运行或已停止。之后,我们将创建一个用于启动任务的按钮。
步骤1:在Android Studio中创建一个新项目
导航到Android Studio,如下面的屏幕所示。在下面的屏幕中,单击“新建项目”以创建一个新的Android Studio项目。
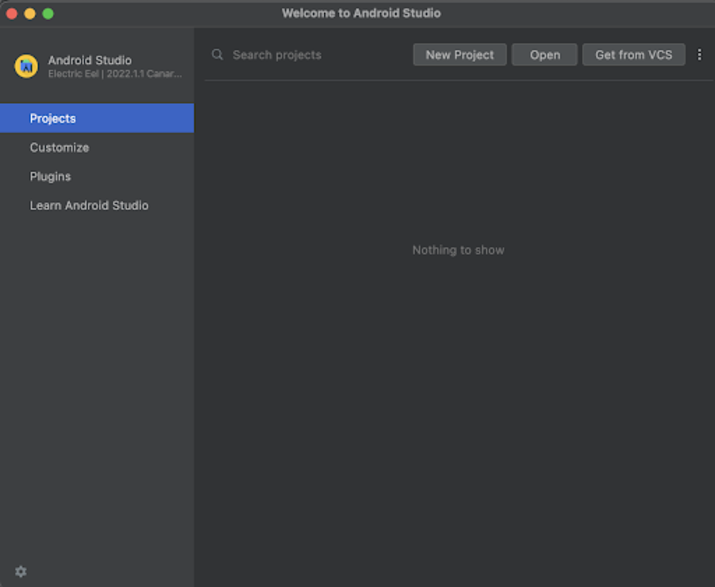
单击“新建项目”后,您将看到下面的屏幕。
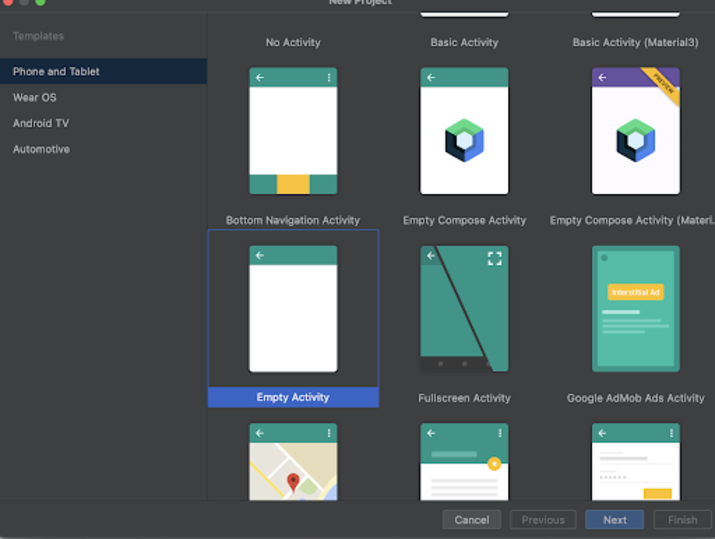
在这个屏幕中,我们只需选择“空活动”,然后单击“下一步”。单击“下一步”后,您将看到下面的屏幕。
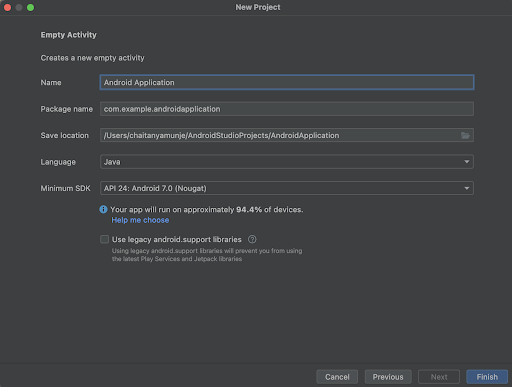
在这个屏幕中,我们只需指定项目名称。然后包名将自动生成。
注意 - 确保选择Java作为语言。
指定所有详细信息后,单击“完成”以创建一个新的Android Studio项目。
项目创建完成后,我们将看到打开的两个文件,即activity_main.xml和MainActivity.java文件。
步骤2:使用activity_main.xml
导航到activity_main.xml。如果此文件不可见,则要打开此文件。在左侧窗格中,导航到app>res>layout>activity_main.xml以打开此文件。打开此文件后,向其中添加以下代码。代码中添加了注释以详细了解。
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <!-- on below line creating a text view for heading --> <TextView android:id="@+id/idTVHeading" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_centerInParent="true" android:layout_margin="5dp" android:padding="4dp" android:text="Returning Boolean from Async Task in Android" android:textAlignment="center" android:textColor="@color/black" android:textSize="20sp" android:textStyle="bold" /> <!-- on below line creating a text view for displaying a message --> <TextView android:id="@+id/idTVMsg" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_below="@id/idTVHeading" android:layout_margin="5dp" android:padding="4dp" android:text="Message" android:textAlignment="center" android:textColor="@color/black" android:textSize="18sp" /> <!-- on below line creating a button to start out task --> <Button android:id="@+id/idBtnStartAsyncTask" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_below="@id/idTVMsg" android:layout_margin="20dp" android:text="Start Asnync Task" android:textAllCaps="false" android:textColor="@color/white" /> </RelativeLayout>
说明:在上面的代码中,我们创建了一个根布局作为相对布局。在这个布局中,我们创建了一个TextView,用于显示应用程序的标题。之后,我们创建了另一个TextView,用于显示我们正在应用程序中执行的当前任务状态。之后,我们创建了一个按钮,我们将使用它来启动任务,任务将运行最多5秒,然后终止。
步骤3:使用MainActivity.java文件
导航到MainActivity.java。如果此文件不可见,则要打开此文件。在左侧窗格中,导航到app>res>layout>MainActivity.java以打开此文件。打开此文件后,向其中添加以下代码。代码中添加了注释以详细了解。
package com.example.java_test_application; import android.os.AsyncTask; import android.os.Bundle; import android.view.View; import android.view.animation.Animation; import android.view.animation.AnimationUtils; import android.widget.Button; import android.widget.RelativeLayout; import android.widget.TextView; import androidx.appcompat.app.AppCompatActivity; public class MainActivity extends AppCompatActivity { // creating variables for button and text view. private TextView msgTV; private Button asyncTaskBtn; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); //initializing variable for text view and button on below line. msgTV = findViewById(R.id.idTVMsg); asyncTaskBtn = findViewById(R.id.idBtnStartAsyncTask); // adding on click listner for button on below line. asyncTaskBtn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // on below line calling our async task manage class AsyncTaskManage asyncTaskManage = new AsyncTaskManage(); // on below line calling execute method to execute it. asyncTaskManage.execute(""); } }); } // on below line creating async task manage class. private class AsyncTaskManage extends AsyncTask{ // on below line creating do in background method. @Override protected Boolean doInBackground(String... strings) { // on below line running try and catch block. try { // on below line sleeping the app by calling sleep method. Thread.sleep(3000); // on below line returning as false. return false; // on below line doing exception handling. } catch (Exception e) { e.printStackTrace(); } // on below line returning as true. return true; } // on below line creating a post execute method. @Override protected void onPostExecute(Boolean aBoolean) { super.onPostExecute(aBoolean); // on below line checking if boolean is true or false. if (aBoolean) { // on below line setting text message as task running. msgTV.setText("Task is running.."); } else { // on below line setting message as task stopped. msgTV.setText("Task is stopped.."); } } } }
说明:在上面的代码中,我们首先为TextView和按钮创建变量。现在我们将看到onCreate方法。这是每个Android应用程序的默认方法。当创建应用程序视图时,将调用此方法。在此方法中,我们设置contentView,即名为activity_main.xml的布局文件,以设置来自该文件UI。在onCreate方法中,我们使用我们在activity_main.xml文件中给出的id来初始化TextView和按钮变量。之后,我们为按钮添加一个点击监听器。在点击监听器方法中,我们调用异步任务并调用execute方法来执行它。之后,我们创建一个名为AsyncTaskManage的类并将其扩展为AsyncTask。在这个类中,我们创建一个在后台执行的方法。在此方法中,我们将任务休眠5秒,在这种情况下,我们返回布尔值false,之后对于默认情况,我们返回true。现在,我们创建另一个方法作为onPostExecute。在此方法中,我们检查从后台执行方法返回的布尔值。如果布尔值为true,我们将在TextView中指定消息为“任务正在运行”,如果布尔值为false,则我们在TextView中指定消息为“任务已停止”。
添加上述代码后,我们只需单击顶部栏中的绿色图标即可在移动设备上运行我们的应用程序。
注意 - 确保已连接到您的真实设备或模拟器。
输出
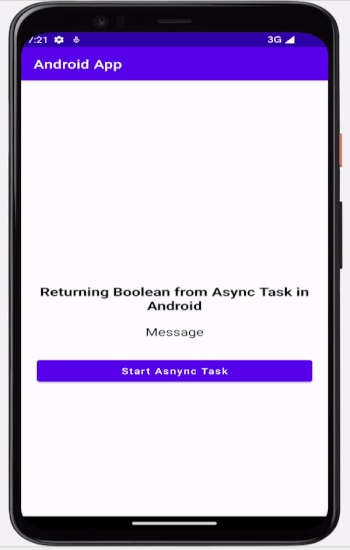
结论
在本文中,我们介绍了如何在Android的AsyncTask中返回布尔值。