如何使用 jQuery 检查数组是否为空?
概述
在 jQuery 中,我们可以使用多种方法轻松检查数组是否为空。常用的方法包括 length 属性和 JavaScript 中的数组是对象,因此可以使用 jQuery 别名符号 $.isEmptyObject(objName)。数组是元素的集合。因此,为了实现我们的目标,我们应该事先了解 jQuery 的语法以及如何操作 HTML。
有必要在 isEmptyObject() 方法中编写 “$”,因为 “$” 是 jQuery 的主要库,其中包含各种方法,如 isEmptyObject()、isArray()、isFunction() 等。在所有这些方法中,都会将对对象的引用作为参数传递,以检查特定方法。
范围
在本文中,我们讨论了以下内容:
各种 jQuery 方法,例如 length 和 isEmptyObject(),
作为参数传递的对象引用。
在 jQuery 函数内部初始化空数组。
语法
在此区域中使用的主要语法:
使用 length 属性
arr.length
使用 isEmptyObject
$.isEmptyObject(object Name)
在 HTML 中编写 jQuery 函数之前,我们应该在 HTML 的 <head> 标签中添加 jQuery 的 cdn 链接。
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.1/jquery.min.js"></script>
方法 01:在此方法中,我们将使用 length 属性来检查数组是否为空。
数组的 length 属性将检查数组的长度并返回一个数字值。数组的索引从 0 开始,但长度从 1 开始计算,因此数组的长度是索引的 +1。当数组不包含任何元素时,它将返回 0,否则它将返回数组的长度。
算法
步骤 1 - 在 HTML 的 body 标签内创建一个 HTML <button>。
步骤 2 - 在 jQuery 语法中传递一个选择器作为按钮,在选定的标签上添加 click() 事件方法操作。
步骤 3 - 创建一个空数组作为 arr[ ]。
步骤 4 - 使用 if-else 条件中的 length 方法检查数组[ ] 的长度。
步骤 5 - 如果数组[ ] 的长度等于零,则它将返回空,否则如果数组的长度大于零,则它将返回空。
示例
<!DOCTYPE html> <html lang="en"> <head> <title>Check array empty or not using jQuery</title> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.1/jquery.min.js"></script> <style> body{ text-align: center; } </style> </head> <body> <p> <strong>Output: </strong> <span id="outputVal" style="padding: 4px;"></span> </p> <button>Check Array</button> <script> $("button").click(() => var arr = []; if(arr.length==0){ document.getElementById("outputVal").innerText="Array is empty"; document.getElementById("outputVal").style.background="tomato"; } else { document.getElementById("outputVal").innerText="Array is not empty"; document.getElementById("outputVal").style.background="lightgreen"; } }) </script> </body> </html>
方法 02:使用 isEmptyObject() 属性
isEmptyObject() 方法检查对象的属性。由于数组在 JavaScript 中是对象,因此数组作为参数通过 isEmptyObject(对象名称) 传递。isEmptyObject 的返回类型是布尔值,因此它将返回 true 或 false。如果传递给它的对象为空,则它将返回 true,否则将返回 false。
算法
步骤 1 - 在 HTML 的 body 标签内创建一个 HTML <button>。
步骤 2 - 在 jQuery 语法中传递一个选择器作为按钮,在选定的标签上添加 click() 事件方法操作。
步骤 3 - 创建一个空数组作为 arr[ ]。
步骤 4 - 使用 isEmptyObject() 方法并将数组作为参数传递给它 isEmptyObject(arr),并将返回值存储在一个变量中。
步骤 5 - 将该变量作为条件传递给 if-else。如果它返回 true,则输出将为数组为空,否则如果它返回 false,则输出将为数组不为空。
示例
<!DOCTYPE html> <html lang="en"> <head> <title>Check array empty or not using jQuery</title> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.1/jquery.min.js"> </script> <style> body{ text-align: center; } </style> </head> <body> <p> <strong>Output: </strong> <span id="outputVal" style="padding: 4px;"></span> </p> <button>Check Array</button> <script> // jQuery starts from here // It is necessary to write the “$” with isEmptyObject() as “$” is the main library of the jQuery which contains this method. $("button").click(() => { var arr = []; var res = $.isEmptyObject(arr); if (res) { document.getElementById("outputVal").innerText = "Array is empty"; document.getElementById("outputVal").style.background = "tomato"; } else { document.getElementById("outputVal").innerText = "Array is not empty"; document.getElementById("outputVal").style.background = "lightgreen"; } }) </script> </body> </html>
以上两种方法的输出相同
当数组为空时
<script> $("button").click(() => { var arr = []; var res = $.isEmptyObject(arr); if (res) { document.getElementById("outputVal").innerText = "Array is empty"; document.getElementById("outputVal").style.background = "tomato"; } else { document.getElementById("outputVal").innerText = "Array is not empty"; document.getElementById("outputVal").style.background = "lightgreen"; } }) </script>
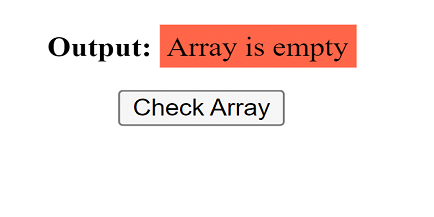
当数组已填充时
<script> $("button").click(() => { var arr = [1,2,3,4]; var res = $.isEmptyObject(arr); if (res) { document.getElementById("outputVal").innerText = "Array is empty"; document.getElementById("outputVal").style.background = "tomato"; } else { document.getElementById("outputVal").innerText = "Array is not empty"; document.getElementById("outputVal").style.background = "lightgreen"; } }) </script>
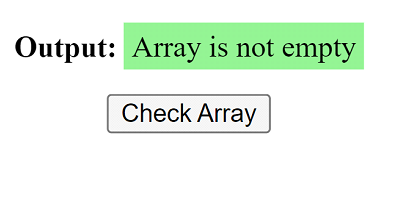
结论
jQuery 简化了 HTML 文档对象模型 (D.O.M.) 中的操作,因为它具有可与数组一起使用的预制属性。jQuery 使代码编写更短、更简单。“isEmptyObject” 存储对象的引用,从中检查空值。除了基本的 if-else 语句之外,我们还可以使用三元运算符。
($.isEmptyObject(arr))? alert(“array is empty”) : alert(“array is not empty”);
它提供单行解决方案而不是多行解决方案。