如何在 ElectronJS 中查找页面上的文本?
概述
页面文本查找功能帮助用户在页面上查找单词。ElectronJS 是一个开源框架,用于创建可在所有操作系统上运行的跨平台桌面应用程序。ElectronJS 具有许多具有各自功能的预定义实例方法。因此,为了构建此页面文本查找功能,ElectronJS 提供了 "findInPage" 方法,该方法获取当前聚焦的窗口并扫描页面上的所有文本。
语法
为了查找页面上的文本,ElectronJS 提供了以下语法来查找文本和清除页面上的搜索结果:
findInPage − 在下面的语法中,“findInPage”是一个方法,它接受文本和选项两个参数。文本是要在页面上查找的单词,另一个参数是根据其扫描页面文本的 findInPage 方法的限制。
currentFocusedWindow − 这是一个当前窗口引用变量,其中 "getFocusedWindow()" 返回要扫描文本的当前窗口。它是 BrowserWindow 对象的方法。
WebContents − 它是一个 ElectronJS 模块,它是一组提供访问权限来操作浏览器窗口的方法。它允许用户更改浏览器页面。
currentFocusedWindow.webContents.findInPage(text, options);
stopFindPage − "stopFindPage" 是 ElectronJS 的一个方法,它通过清除页面上突出显示的单词来停止 findInPage 过程,从而清除页面。stopFindInPage 也接受一个名为 "clearSelection" 的参数作为输入,此参数传递给方法以清除页面突出显示。
currentFocusedWindow.webContents.stopFindInPage('clearSelection');
算法
步骤 1 − 创建一个名为 "electronApp" 的文件夹,并在文本编辑器(例如 VS Code)中打开该文件夹。
步骤 2 − 现在打开代码编辑器的集成终端或打开您的 PowerShell。
步骤 3 − 使用以下命令检查您的系统是否已安装 Node。如果没有,请访问 NodeJS 官方网站并下载适合您系统的版本。
node --version
步骤 4 − 现在使用下面显示的命令创建一个 'package.json' 文件。
npm init
步骤 5 − 现在使用以下命令将 Electron 依赖项安装到 package.json 文件中。
npm install electron –-save
步骤 6 − 安装后,在文件夹中创建一个 "main.js" 文件,并使用以下代码创建一个浏览器窗口,"main.js" 是桌面应用程序的入口点。还要检查 package.json 文件,并将 main 设置为 "main.js"。
const { app, BrowserWindow } = require('electron') var win; function createWindow() { win = new BrowserWindow({ width: 800, height: 600, webPreferences: { nodeIntegration: true } }); win.loadFile('src/index.html') win.webContents.openDevTools() } app.whenReady().then(() => { createWindow() }) app.on('window-all-closed', () => { if (process.platform !== 'darwin') { app.quit() } }) app.on('activate', () => { if (BrowserWindow.getAllWindows().length === 0) { createWindow() } })
步骤 7 − 现在在父文件夹内创建一个 src 文件夹,并在其中创建两个文件 "index.html" 和 "index.js"。
步骤 8 − 打开 index.html 文件,并将 HTML 模板添加到该文件中。
步骤 9 − 现在将元标记添加到文件的 head 标记中,该标记为页面添加 "内容安全策略"。
<meta http-equiv="Content-Security-Policy" content="script-src 'self' 'unsafe-inline';" />
步骤 10 − 现在创建一个 div 容器,其中包含一个输入标记和两个按钮,其值和 ID 名称分别为 "search" 和 "clearSearch"。
<div id="searchPannel"> <input id="enter" type="text" placeholder="type word to search..."> <button id="search">Find in Text</button> <button id="clearSearch">Clear Selection</button> </div>
步骤 11 − 现在创建另一个 div 容器,其中包含用于搜索的示例文本。
<div> This is a sample text to search for any word. Tutorialspoint is a great platform which provides information regarding each and every domain. We also provide training courses to level up your skills. Vist tutorialspoint.com to get more information about our platform. Also you can read articles regarding any topic. </div>
步骤 12 − 现在将 "index.js" 链接到 HTML 文件,其中包含在页面上搜索文本的功能。
<script src="index.js"></script>
步骤 13 − 现在打开 index.js 文件并从 Electron 模块导入 "BrowserWindow"。
const electron = require('electron') const BrowserWindow = electron.remote.BrowserWindow;
步骤 14 − 现在创建一个名为 "option" 的对象,其中包含一些查找文本的约束条件。
var options = { forward: true, findNext: false, matchCase: false, wordStart: false, medialCapitalAsWordStart: false }
步骤 15 − 现在通过 HTML DOM 访问 "find" 按钮和 "clear" 按钮到引用变量。
var search = document.getElementById('search'); var clearSearch = document.getElementById('clearSearch');
步骤 16 − 现在使用 addEventListener 结合 click 事件监听器在页面上查找文本。
search.addEventListener('click', () => { const cWin = BrowserWindow.getFocusedWindow(); var text = document.getElementById('enter').value; console.log(text); if (text) { const requestId = cWin.webContents.findInPage(text, options); console.log(requestId); } else { console.log('Enter Text to find'); } cWin.webContents.on('found-in-page', (event, result) => { console.log(result.requestId); console.log(result.activeMatchOrdinal); console.log(result.matches); console.log(result.selectionArea); }); });
步骤 17 − 现在使用 "clear" addEventListener 结合 click 事件监听器停止在页面上查找文本的过程。
clearSearch.addEventListener('click', () => { const cWin = BrowserWindow.getFocusedWindow(); cWin.webContents.stopFindInPage('clearSelection'); console.log("Search cleared"); });
步骤 18 − 现在检查 package.json 文件,并在 "script" 中设置以下键值对。
"scripts": { "start": "electron ." }
步骤 19 − 现在使用 "npm start" 命令启动桌面应用程序。
示例
在这个例子中,我们将使用 ElectronJS 创建一个桌面应用程序。这个桌面应用程序将具有一个功能,可以在当前窗口页面上搜索任何文本。为了构建此功能,我们将在 Electron 应用程序的渲染进程中使用 "webContents" 模块 "findInPage" 和 "stopFindPage" 方法。为此,我们将在 main.js 中创建 Electron 应用程序的主进程,在 index.js 中创建渲染器进程。index.html 将包含页面的前端。
main.js
const { app, BrowserWindow } = require('electron') var win; function createWindow() { win = new BrowserWindow({ width: 800, height: 600, webPreferences: { nodeIntegration: true } }); win.loadFile('src/index.html') win.webContents.openDevTools() } app.whenReady().then(() => { createWindow() }) app.on('window-all-closed', () => { if (process.platform !== 'darwin') { app.quit() } }) app.on('activate', () => { if (BrowserWindow.getAllWindows().length === 0) { createWindow() } })
index.html
<html> <head> <meta http-equiv="Content-Security-Policy" content="script-src 'self' 'unsafe-inline';" /> <title> find text on page using electronjs</title> <style> body{ background-color: white; color: black; } </style> </head> <body> <div id="searchPannel"> <input id="enter" type="text" placeholder="type word to search..."> <button id="search">Find</button> <button id="clearSearch">Clear</button> </div> <hr> <div> This is a sample text to search any word. Tutorialspoint is a great platform which provides information regarding each and every domain. We also provide training courses to level up your skills. Vist tutorialspoint.com to get more information about our platform. Also you can read articles regarding any topic. </div> <script src="index.js"></script> </body> </html>
index.js
const electron = require('electron') const BrowserWindow = electron.remote.BrowserWindow; var options = { forward: true, findNext: false, matchCase: false, wordStart: false, medialCapitalAsWordStart: false } var search = document.getElementById('search'); var clearSearch = document.getElementById('clearSearch'); search.addEventListener('click', () => { const cWin = BrowserWindow.getFocusedWindow(); var text = document.getElementById('enter').value; console.log(text); if (text) { const requestId = cWin.webContents.findInPage(text, options); console.log(requestId); } else { console.log('Enter Text to find'); } cWin.webContents.on('found-in-page', (event, result) => { console.log(result.requestId); console.log(result.activeMatchOrdinal); console.log(result.matches); console.log(result.selectionArea); }); }); clearSearch.addEventListener('click', () => { const cWin = BrowserWindow.getFocusedWindow(); cWin.webContents.stopFindInPage('clearSelection'); console.log("Search cleared"); });
输出
在下图中,当用户启动应用程序时,他将获得一个界面,该界面包含一个输入框(用于接收用户的输入)和一个搜索按钮、清除按钮以及它们各自的功能。当用户在输入按钮中键入文本并点击 "查找" 按钮时,将触发 "findInPage" 方法,并通过突出显示屏幕上的文本(如第二张图像所示)来输出结果。当用户点击清除按钮时,文本中的所有突出显示都将被移除。
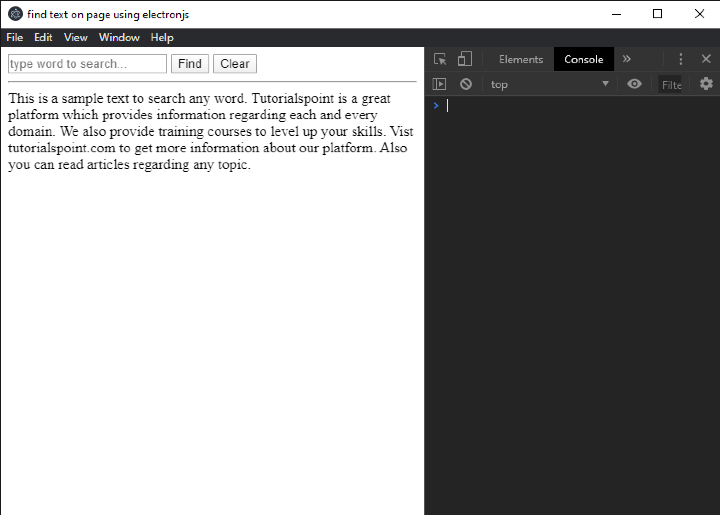
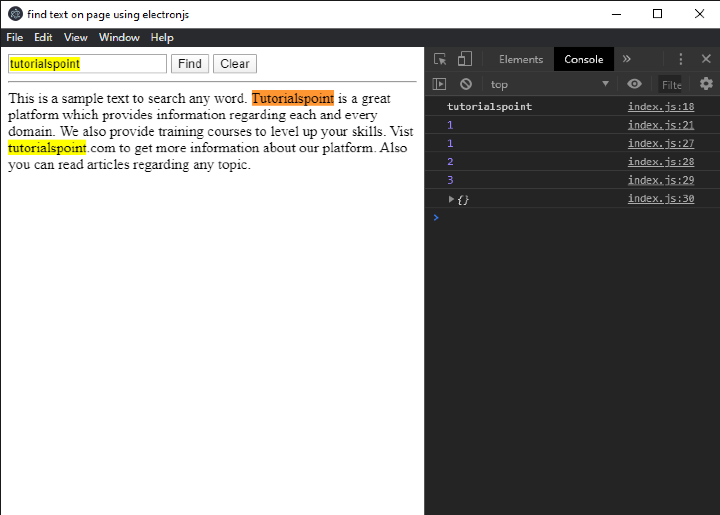
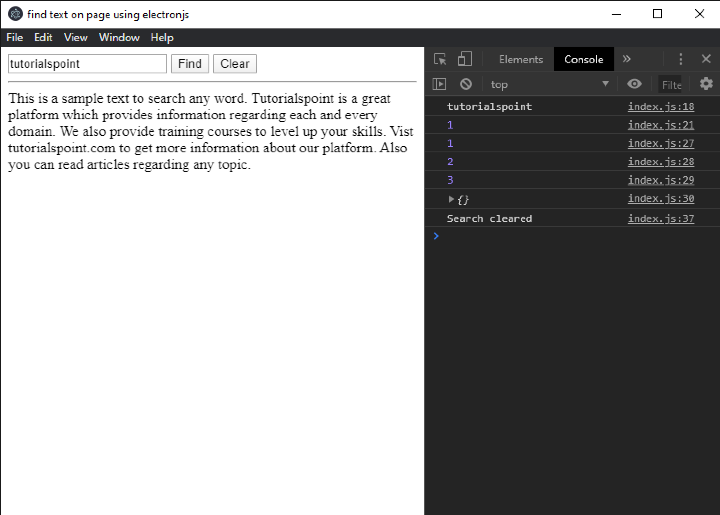
结论
当页面上存在大量内容时,此功能非常有助于查找页面上的文本。在上图中,我们可以看到控制台中有一些数字。它们是请求次数,表示用户请求文本的次数。当在页面上找到文本时,所有返回的文本都将以不同的颜色显示,表示文本出现的次数。在清除函数中,确保必须传递 "clearSelection" 作为参数,否则您将收到错误消息。