如何在 Tkinter 应用程序中监听终端?
将终端的功能与 Tkinter 应用程序结合起来可以增强其功能和多功能性。在本教程中,我们将探讨如何使用 Python 的subprocess 模块将终端功能集成到 Tkinter 应用程序中,并提供一个实际示例。
要理解集成过程,必须清楚地了解所涉及的核心组件:
Tkinter − Tkinter 是 Python 事实上的 GUI 工具包,它为开发人员提供了构建图形应用程序的全面工具和组件。
subprocess 模块 − Python 的 subprocess 模块对于创建附加进程、管理其输入/输出/错误管道以及检索返回码至关重要。此模块构成了在 Python 脚本中执行终端命令的基础。
实现示例
让我们检查一个 Python 脚本,该脚本举例说明了将终端功能集成到 Tkinter 应用程序中的方法。此脚本创建一个基本的应用程序,该应用程序执行“help”命令并在 Tkinter 窗口中显示其输出。
脚本的分步实现如下:
步骤 1:类初始化
def __init__(self, root): self.root = root self.root.title("Terminal App")
__init__ 方法初始化 Tkinter 窗口并设置其标题,从而建立应用程序的入口点。
步骤 2:用于输出的文本小部件
self.output_text = tk.Text(self.root, wrap="word", height=20, width=50) self.output_text.pack(padx=10, pady=10)
创建一个 Tkinter Text 小部件来显示终端输出。wrap="word" 选项确保在单词边界处正确换行,从而提高视觉清晰度。然后将小部件打包到窗口中,并指定填充。
步骤 3:用于命令执行的按钮
self.execute_button = tk.Button(self.root, text="Run Command", command=self.run_command) self.execute_button.pack(pady=10)
向窗口添加一个标记为“运行命令”的 Tkinter Button。按下此按钮时,将调用 run_command 方法。
步骤 4:执行命令
def run_command(self): command = "help" result = subprocess.run(command, shell=True, capture_output=True, text=True) self.output_text.delete("1.0", tk.END) self.output_text.insert(tk.END, result.stdout) self.output_text.insert(tk.END, result.stderr)
run_command 方法定义终端命令(在本例中为“help”),并使用 subprocess.run() 执行它。捕获标准输出 (stdout) 和标准错误 (stderr),并在 Tkinter Text 小部件中显示它们。
完整代码
让我们结合这些步骤并检查完整的实现示例:
示例
import tkinter as tk import subprocess class TerminalApp: def __init__(self, root): self.root = root self.root.title("Listening to Terminal on Tkinter App") root.geometry("720x400") # Create a Text widget to display the terminal output self.output_text = tk.Text(self.root, wrap="word", height=20, width=50) self.output_text.pack(padx=10, pady=10) # Create a button to execute a command self.execute_button = tk.Button(self.root, text="Run Command", command=self.run_command) self.execute_button.pack(pady=10) def run_command(self): # The command you want to execute command = "help" # Use subprocess to run the command and capture the output result = subprocess.run(command, shell=True, capture_output=True, text=True) # Display the output in the Text widget self.output_text.delete("1.0", tk.END) # Clear previous output self.output_text.insert(tk.END, result.stdout) self.output_text.insert(tk.END, result.stderr) if __name__ == "__main__": root = tk.Tk() app = TerminalApp(root) root.mainloop()
输出
运行上述示例后,您将看到一个带有“运行命令”按钮的 Tkinter 窗口。单击按钮后,它将运行特定命令并在 Tkinter 窗口中显示结果。(在我们的示例中,它将运行“help”命令)。
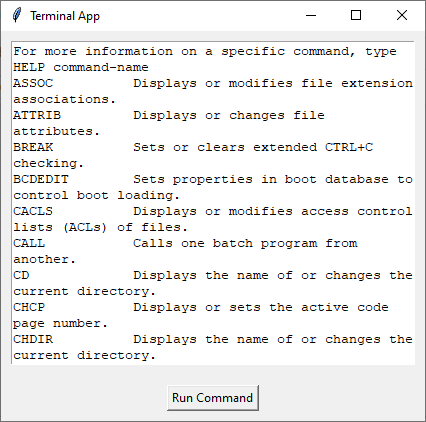
结论
将终端功能集成到 Tkinter 应用程序中,通过在应用程序界面中启用直接系统交互来扩展用户体验。