如何使用 Python tkSimpleDialog.askstring?
在 Python 中,Tkinter 库提供了一套强大的工具来构建 GUI,而 "tkSimpleDialog.askstring" 就是其中一个工具,它可以通过简单的对话框来获取用户输入。
了解 Tkinter 和简单对话框
Tkinter 是 Python 的标准 GUI 工具包,它允许开发人员轻松创建桌面应用程序。它提供各种小部件,包括按钮、标签和输入字段,以构建交互式界面。Tkinter 的 tkSimpleDialog 模块专门专注于提供简单的对话框以进行用户交互,而 askstring 是它提供的函数之一。
开始使用 tkSimpleDialog.askstring
askstring 函数旨在通过对话框提示用户输入字符串。让我们从创建一个基本的 Tkinter 窗口并使用 askstring 获取用户输入开始 -
示例
import tkinter as tk from tkinter import simpledialog # Create the main Tkinter window root = tk.Tk() root.title("tkSimpleDialog.askstring Example") root.geometry("720x250") # Function to show the string input dialog def get_string_input(): result = simpledialog.askstring("Input", "Enter a string:") if result: # Do something with the entered string print("Entered string:", result) # Create a button that, when clicked, calls the get_string_input function button = tk.Button(root, text="Get String Input", command=get_string_input) button.pack(pady=20) # Start the Tkinter event loop root.mainloop()
输出
此代码设置了一个带有按钮的基本 Tkinter 窗口。当点击按钮时,会调用 get_string_input 函数,该函数依次使用 askstring 显示带有指定提示(“输入字符串:”)的对话框。然后将输入的字符串打印到控制台。
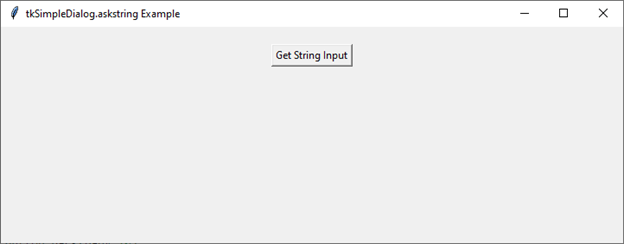
自定义对话框
askstring 函数允许您自定义对话框的各个方面,例如标题、提示和初始值。让我们探讨如何自定义这些参数 -
示例
import tkinter as tk from tkinter import simpledialog # Create the main Tkinter window root = tk.Tk() root.title("Customizing tkSimpleDialog.askstring") root.geometry("720x250") # Function to show the customized string input dialog def get_custom_string_input(): result = simpledialog.askstring( "Custom Input", "Enter your name:", initialvalue="TutorialsPoint.com" ) if result: print("Entered name:", result) # Create a button to call the get_custom_string_input function button = tk.Button( root, text="Get Custom String Input", command=get_custom_string_input ) button.pack(pady=20) # Start the Tkinter event loop root.mainloop()
在此示例中,askstring 函数使用标题(“自定义输入”)、提示(“输入您的姓名:”)和初始值(“John Doe”)进行了自定义。这些自定义使对话框更符合应用程序的上下文。
输出
运行此代码后,您将获得以下输出窗口 -
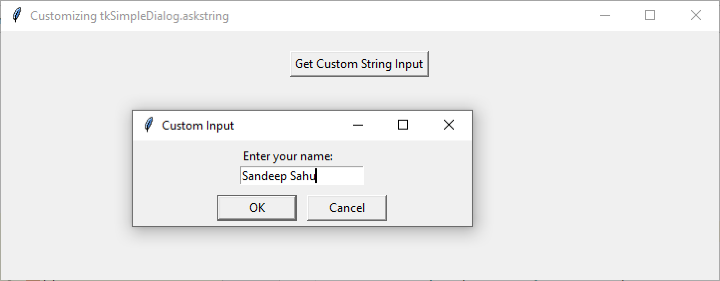
提供自定义字符串输入后,您可以将其打印到控制台 -
Entered name: Sandeep Sahu
tkSimpleDialog.askstring 的实际应用
现在我们已经基本了解了如何使用 askstring,让我们探讨一下此函数的一些实际应用。
收集用户凭据
请看以下示例 -
示例
import tkinter as tk from tkinter import simpledialog # Create the main Tkinter window root = tk.Tk() root.title("User Credentials Example") root.geometry("720x250") # Function to get username and password def get_credentials(): username = simpledialog.askstring( "Login", "Enter your username:" ) if username: password = simpledialog.askstring( "Login", "Enter your password:", show="*" ) if password: # Do something with the entered username and password print("Username:", username) print("Password:", password) # Create a button to call the get_credentials function button = tk.Button(root, text="Login", command=get_credentials) button.pack(pady=20) # Start the Tkinter event loop root.mainloop()
在此示例中,askstring 函数用于收集用户名和密码。show="*" 参数用于隐藏输入的密码字符,增强安全性。
输出
运行此代码后,您将获得以下输出窗口 -
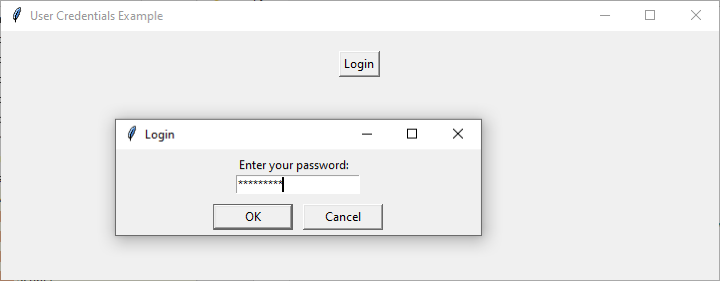
修改凭据后,您可以将修改后的详细信息打印到控制台 -
Username: Arun Kumar Password: HelloArun
接收用户首选项或设置
请看以下示例 -
示例
import tkinter as tk from tkinter import simpledialog root = tk.Tk() root.title("User Preferences Example") root.geometry("720x250") # Function to get user preferences def get_preferences(): # Get user's favorite color color = simpledialog.askstring( "Preferences", "Enter your favorite color:", initialvalue="Blue" ) if color: # Get user's preferred font size font_size = simpledialog.askstring( "Preferences", "Enter your preferred font size:", initialvalue="12" ) if font_size: # Do something with the entered preferences print("Favorite Color:", color) print("Preferred Font Size:", font_size) # Create a button that, when clicked, calls the get_preferences function button = tk.Button(root, text="Set Preferences", command=get_preferences) button.pack(pady=20) root.mainloop()
此示例演示了如何使用 askstring 收集用户首选项,例如他们喜欢的颜色和首选字体大小。
输出
运行此代码后,您将获得以下输出窗口 -
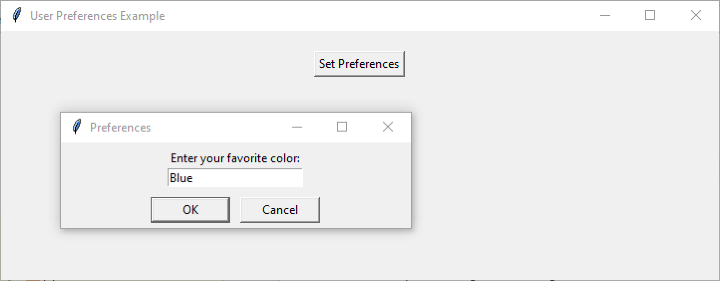
设置首选项后,您将在控制台上打印修改后的设置 -
Favorite Color: Blue Preferred Font Size: 20
结论
在本教程中,我们探讨了 Python Tkinter 中 "tkSimpleDialog.askstring" 的用法。我们从基础开始,了解如何创建简单的字符串输入对话框,并逐步转向自定义选项。
通过提供实际示例,我们展示了askstring在收集各种类型用户输入方面的多功能性,从简单的字符串到敏感信息(如密码)。